Commit 25ef43892ed984c1f8eba8ff7e1b1558a12802b8
1 parent
4dd5e233
Restructuring repo to match branches/tags/trunk.
Showing
100 changed files
with
3645 additions
and
0 deletions
Too many changes to show.
To preserve performance only 100 of 100+ files are displayed.
samples/coordinatesGrid-sample/pom.xml
0 → 100644
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
3 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
4 | + <parent> | ||
5 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
6 | + <artifactId>parent</artifactId> | ||
7 | + <version>3.3.4-SNAPSHOT</version> | ||
8 | + <relativePath>../pom.xml</relativePath> | ||
9 | + </parent> | ||
10 | + <modelVersion>4.0.0</modelVersion> | ||
11 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
12 | + <artifactId>coordinatesGrid-sample</artifactId> | ||
13 | + <packaging>war</packaging> | ||
14 | + <name>coordinatesGrid-sample Maven Webapp</name> | ||
15 | + <build> | ||
16 | + <finalName>${project.artifactId}</finalName> | ||
17 | + </build> | ||
18 | + <dependencies> | ||
19 | + <dependency> | ||
20 | + <groupId>org.richfaces.ui</groupId> | ||
21 | + <artifactId>richfaces-ui</artifactId> | ||
22 | + </dependency> | ||
23 | + <dependency> | ||
24 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
25 | + <artifactId>coordinatesGrid</artifactId> | ||
26 | + <version>${project.version}</version> | ||
27 | + </dependency> | ||
28 | + <dependency> | ||
29 | + <groupId>com.uwyn</groupId> | ||
30 | + <artifactId>jhighlight</artifactId> | ||
31 | + <version>1.0</version> | ||
32 | + </dependency> | ||
33 | + </dependencies> | ||
34 | +</project> |
samples/coordinatesGrid-sample/src/main/java/org/richfaces/sandbox/coordinatesGrid/Seat.java
0 → 100644
1 | +package org.richfaces.sandbox.coordinatesGrid; | ||
2 | + | ||
3 | +import java.io.Serializable; | ||
4 | + | ||
5 | +/** | ||
6 | + * @author a180963 | ||
7 | + */ | ||
8 | +public class Seat implements Serializable { | ||
9 | + | ||
10 | + private int row; | ||
11 | + private int seatNr; | ||
12 | + private boolean enabled; | ||
13 | + | ||
14 | + public int getRow() { | ||
15 | + return row; | ||
16 | + } | ||
17 | + | ||
18 | + public void setRow(int row) { | ||
19 | + this.row = row; | ||
20 | + } | ||
21 | + | ||
22 | + public int getSeatNr() { | ||
23 | + return seatNr; | ||
24 | + } | ||
25 | + | ||
26 | + public void setSeatNr(int seatNr) { | ||
27 | + this.seatNr = seatNr; | ||
28 | + } | ||
29 | + | ||
30 | + public boolean isEnabled() { | ||
31 | + return enabled; | ||
32 | + } | ||
33 | + | ||
34 | + public void setEnabled(boolean enabled) { | ||
35 | + this.enabled = enabled; | ||
36 | + } | ||
37 | +} |
samples/coordinatesGrid-sample/src/main/java/org/richfaces/sandbox/coordinatesGrid/SeatSelector.java
0 → 100644
1 | +package org.richfaces.sandbox.coordinatesGrid; | ||
2 | + | ||
3 | +import org.ajax4jsf.model.DataVisitor; | ||
4 | +import org.ajax4jsf.model.Range; | ||
5 | +import org.ajax4jsf.model.SequenceDataModel; | ||
6 | +import org.ajax4jsf.model.SequenceRange; | ||
7 | +import org.richfaces.component.event.CoordinatesSelectionEvent; | ||
8 | +import org.richfaces.component.model.SquareRange; | ||
9 | + | ||
10 | +import javax.faces.application.FacesMessage; | ||
11 | +import javax.faces.context.FacesContext; | ||
12 | +import javax.faces.model.DataModel; | ||
13 | +import javax.faces.model.ListDataModel; | ||
14 | +import java.io.IOException; | ||
15 | +import java.util.AbstractList; | ||
16 | + | ||
17 | +public class SeatSelector { | ||
18 | + | ||
19 | + private Integer rows = 10; | ||
20 | + private Integer cols = 20; | ||
21 | + private Integer firstRow = 0; | ||
22 | + private Integer firstCol = 0; | ||
23 | + private Seat[][] seats = new Seat[rows][cols]; | ||
24 | + private DataModel dataModel = new CustomDataModel(); | ||
25 | + | ||
26 | + public SeatSelector() { | ||
27 | + | ||
28 | + final Seat seat = new Seat(); | ||
29 | + seat.setEnabled(true); | ||
30 | + seat.setRow(3); | ||
31 | + seat.setSeatNr(5); | ||
32 | + seats[3][4] = seat; | ||
33 | + } | ||
34 | + | ||
35 | + public Integer getRows() { | ||
36 | + return rows; | ||
37 | + } | ||
38 | + | ||
39 | + public void setRows(Integer rows) { | ||
40 | + this.rows = rows; | ||
41 | + resizeSeats(); | ||
42 | + } | ||
43 | + | ||
44 | + public Integer getCols() { | ||
45 | + return cols; | ||
46 | + } | ||
47 | + | ||
48 | + public void setCols(Integer cols) { | ||
49 | + this.cols = cols; | ||
50 | + resizeSeats(); | ||
51 | + } | ||
52 | + | ||
53 | + public Integer getFirstRow() { | ||
54 | + return firstRow; | ||
55 | + } | ||
56 | + | ||
57 | + public void setFirstRow(Integer row) { | ||
58 | + this.firstRow = row; | ||
59 | + } | ||
60 | + | ||
61 | + public Integer getFirstCol() { | ||
62 | + return firstCol; | ||
63 | + } | ||
64 | + | ||
65 | + public void setFirstCol(Integer col) { | ||
66 | + this.firstCol = col; | ||
67 | + } | ||
68 | + | ||
69 | + public DataModel getDataModel() { | ||
70 | + return dataModel; | ||
71 | + } | ||
72 | + | ||
73 | + public void itemsSelected(CoordinatesSelectionEvent e) { | ||
74 | + for (int y = e.getStartY(); y <= e.getEndY(); y++) { | ||
75 | + for (int x = e.getStartX(); x <= e.getEndX(); x++) { | ||
76 | + if (seats[y][x] == null) { | ||
77 | + Seat seat = new Seat(); | ||
78 | + seat.setRow(y); | ||
79 | + seat.setSeatNr(x); | ||
80 | + seat.setEnabled(true); | ||
81 | + seats[y][x] = seat; | ||
82 | + } else { | ||
83 | + seats[y][x] = null; | ||
84 | + } | ||
85 | + } | ||
86 | + } | ||
87 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(e.toString())); | ||
88 | + } | ||
89 | + | ||
90 | + public void itemsReserved(CoordinatesSelectionEvent e) { | ||
91 | + for (int y = e.getStartY(); y <= e.getEndY(); y++) { | ||
92 | + for (int x = e.getStartX(); x <= e.getEndX(); x++) { | ||
93 | + if (seats[y][x] != null) { | ||
94 | + seats[y][x].setEnabled(!seats[y][x].isEnabled()); | ||
95 | + } | ||
96 | + } | ||
97 | + } | ||
98 | + } | ||
99 | + | ||
100 | + private void resizeSeats() { | ||
101 | + Seat[][] newSeats = new Seat[rows][cols]; | ||
102 | + for (int y = 0; y < Math.min(seats.length, newSeats.length); y++) { | ||
103 | + System.arraycopy(seats[y], 0, newSeats[y], 0, Math.min(seats[y].length, newSeats[y].length)); | ||
104 | + } | ||
105 | + seats = newSeats; | ||
106 | + } | ||
107 | + | ||
108 | + private class CustomDataModel extends SequenceDataModel { | ||
109 | + | ||
110 | + public CustomDataModel() { | ||
111 | + super(new ListDataModel(new AbstractList<Seat>() { | ||
112 | + | ||
113 | + @Override | ||
114 | + public Seat get(int index) { | ||
115 | + int y = index / cols; | ||
116 | + int x = index % cols; | ||
117 | + return seats[y][x]; | ||
118 | + } | ||
119 | + | ||
120 | + @Override | ||
121 | + public int size() { | ||
122 | + return cols * rows; | ||
123 | + } | ||
124 | + })); | ||
125 | + } | ||
126 | + | ||
127 | + @Override | ||
128 | + public boolean isRowAvailable() { | ||
129 | + return super.isRowAvailable() && getRowData() != null; | ||
130 | + } | ||
131 | + | ||
132 | + @Override | ||
133 | + public void walk(FacesContext context, DataVisitor visitor, Range range, Object argument) throws IOException { | ||
134 | + SquareRange squareRange = null; | ||
135 | + if (range instanceof SquareRange) { | ||
136 | + squareRange = (SquareRange) range; | ||
137 | + } | ||
138 | + if (squareRange == null) { | ||
139 | + super.walk(context, visitor, new SequenceRange(0, -1), argument); | ||
140 | + } else { | ||
141 | + for (int y = squareRange.getStartY(); y <= squareRange.getEndY(); y++) { | ||
142 | + for (int x = squareRange.getStartX(); x <= squareRange.getEndX(); x++) { | ||
143 | + if (0 <= y && y < seats.length && 0 <= x && x < seats[y].length && seats[y][x] != null) { | ||
144 | + visitor.process(context, y * cols + x, argument); | ||
145 | + } | ||
146 | + } | ||
147 | + } | ||
148 | + } | ||
149 | + } | ||
150 | + } | ||
151 | +} |
1 | +<?xml version="1.0"?> | ||
2 | +<!DOCTYPE faces-config PUBLIC "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" | ||
3 | + "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> | ||
4 | +<faces-config> | ||
5 | + <managed-bean> | ||
6 | + <managed-bean-name>seatSelector</managed-bean-name> | ||
7 | + <managed-bean-class>org.richfaces.sandbox.coordinatesGrid.SeatSelector</managed-bean-class> | ||
8 | + <managed-bean-scope>session</managed-bean-scope> | ||
9 | + </managed-bean> | ||
10 | +</faces-config> | ||
11 | + |
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
3 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
4 | + <description>Richfaces coordinatesGrid-sm Sample</description> | ||
5 | + <display-name>coordinatesGrid-sm-sample</display-name> | ||
6 | + <context-param> | ||
7 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
8 | + <param-value>.xhtml</param-value> | ||
9 | + </context-param> | ||
10 | + <context-param> | ||
11 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
12 | + <param-value>2</param-value> | ||
13 | + </context-param> | ||
14 | + <context-param> | ||
15 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
16 | + <param-value>true</param-value> | ||
17 | + </context-param> | ||
18 | + <context-param> | ||
19 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
20 | + <param-value>server</param-value> | ||
21 | + </context-param> | ||
22 | + <context-param> | ||
23 | + <param-name>com.sun.faces.validateXml</param-name> | ||
24 | + <param-value>true</param-value> | ||
25 | + </context-param> | ||
26 | + <context-param> | ||
27 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
28 | + <param-value>false</param-value> | ||
29 | + </context-param> | ||
30 | + <context-param> | ||
31 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
32 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
33 | + </context-param> | ||
34 | + <context-param> | ||
35 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
36 | + <param-value>false</param-value> | ||
37 | + </context-param> | ||
38 | + <context-param> | ||
39 | + <param-name>org.richfaces.SKIN</param-name> | ||
40 | + <param-value>blueSky</param-value> | ||
41 | + </context-param> | ||
42 | + <context-param> | ||
43 | + <param-name>org.richfaces.CONTROL_SKINNING_LEVEL</param-name> | ||
44 | + <param-value>extended</param-value> | ||
45 | + </context-param> | ||
46 | + <filter> | ||
47 | + <display-name>Ajax4jsf Filter</display-name> | ||
48 | + <filter-name>ajax4jsf</filter-name> | ||
49 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
50 | + <init-param> | ||
51 | + <param-name>enable-cache</param-name> | ||
52 | + <param-value>false</param-value> | ||
53 | + </init-param> | ||
54 | + </filter> | ||
55 | + <filter-mapping> | ||
56 | + <filter-name>ajax4jsf</filter-name> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <dispatcher>FORWARD</dispatcher> | ||
59 | + <dispatcher>REQUEST</dispatcher> | ||
60 | + <dispatcher>INCLUDE</dispatcher> | ||
61 | + </filter-mapping> | ||
62 | + <servlet> | ||
63 | + <servlet-name>Faces Servlet</servlet-name> | ||
64 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
65 | + <load-on-startup>1</load-on-startup> | ||
66 | + </servlet> | ||
67 | + <servlet-mapping> | ||
68 | + <servlet-name>Faces Servlet</servlet-name> | ||
69 | + <url-pattern>*.jsf</url-pattern> | ||
70 | + </servlet-mapping> | ||
71 | + <login-config> | ||
72 | + <auth-method>BASIC</auth-method> | ||
73 | + </login-config> | ||
74 | +</web-app> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" | ||
4 | + xmlns:f="http://java.sun.com/jsf/core" | ||
5 | + xmlns:h="http://java.sun.com/jsf/html" xmlns:a4j="http://richfaces.org/a4j" xmlns:rich="http://richfaces.org/rich" | ||
6 | + xmlns:coordinatesGrid="http://richfaces.org/sandbox/coordinatesGrid" | ||
7 | + xmlns:notify="http://richfaces.org/sandbox/notify"> | ||
8 | +<head> | ||
9 | + <title>CoordinatesGrid sample</title> | ||
10 | + <style type="text/css"> | ||
11 | + .rf-cg td.enabled { | ||
12 | + background-color: #aaa; | ||
13 | + } | ||
14 | + | ||
15 | + .rf-cg td.disabled { | ||
16 | + background-color: inherit; | ||
17 | + } | ||
18 | + | ||
19 | + .booker td.enabled { | ||
20 | + background-color: #dfd; | ||
21 | + } | ||
22 | + | ||
23 | + .booker td.disabled { | ||
24 | + background-color: #ddd; | ||
25 | + } | ||
26 | + | ||
27 | + .rf-cg td.highlight { | ||
28 | + background-color: #{a4jSkin.headerBackgroundColor}; | ||
29 | + } | ||
30 | + </style> | ||
31 | + <script type="text/javascript"> | ||
32 | + function selectHandler(startX, startY, endX, endY) { | ||
33 | + jQuery("#status").html(startX + ";" + startY + ";" + endX + ";" + endY); | ||
34 | + } | ||
35 | + function clickHandler(x, y) { | ||
36 | + jQuery("#status").html("click:" + x + ";" + y); | ||
37 | + } | ||
38 | + </script> | ||
39 | +</head> | ||
40 | + | ||
41 | +<body class="rich-container"> | ||
42 | +<ui:include src="menu.xhtml"/> | ||
43 | + | ||
44 | +<p> | ||
45 | + | ||
46 | +</p> | ||
47 | +<notify:notifyMessages ajaxRendered="true"/> | ||
48 | +<h:panelGrid columns="3"> | ||
49 | + <h:panelGroup> | ||
50 | + <h:form> | ||
51 | + <h:panelGrid columns="2"> | ||
52 | + <h:outputLabel value="rows" for="rows"/> | ||
53 | + <h:inputText value="#{seatSelector.rows}" id="rows"/> | ||
54 | + <h:outputLabel value="cols" for="cols"/> | ||
55 | + <h:inputText value="#{seatSelector.cols}" id="cols"/> | ||
56 | + </h:panelGrid> | ||
57 | + <h:commandButton value="Submit"/> | ||
58 | + </h:form> | ||
59 | + | ||
60 | + </h:panelGroup> | ||
61 | + | ||
62 | +</h:panelGrid> | ||
63 | +<h:form> | ||
64 | + <h:panelGrid columns="2"> | ||
65 | + <coordinatesGrid:coordinatesGrid | ||
66 | + selectable="true" | ||
67 | + value="#{seatSelector.dataModel}" | ||
68 | + var="seat" | ||
69 | + rows="#{seatSelector.rows}" | ||
70 | + cols="#{seatSelector.cols}" | ||
71 | + onselect="selectHandler(startX,startY,endX,endY);" | ||
72 | + onclick="clickHandler(x,y);" | ||
73 | + selectionListener="#{seatSelector.itemsSelected}" | ||
74 | + rowIndexVar="rowIndex" | ||
75 | + rowHeaderText="#{rowIndex+1}" | ||
76 | + rowHeaderPosition="both" | ||
77 | + colIndexVar="colIndex" | ||
78 | + colHeaderText="#{colIndex+1}" | ||
79 | + colHeaderPosition="both" | ||
80 | + reRender="booker" | ||
81 | + > | ||
82 | + <coordinatesGrid:coordinatesGridItem x="#{seat.seatNr}" y="#{seat.row}" text="#{seat.row}x#{seat.seatNr}" | ||
83 | + styleClass="enabled"/> | ||
84 | + </coordinatesGrid:coordinatesGrid> | ||
85 | + <coordinatesGrid:coordinatesGrid id="booker" styleClass="booker" | ||
86 | + value="#{seatSelector.dataModel}" | ||
87 | + var="seat" | ||
88 | + rows="#{seatSelector.rows}" | ||
89 | + cols="#{seatSelector.cols}" | ||
90 | + onselect="selectHandler(startX,startY,endX,endY);" | ||
91 | + onclick="clickHandler(x,y);" | ||
92 | + selectionListener="#{seatSelector.itemsReserved}" | ||
93 | + rowIndexVar="rowIndex" | ||
94 | + rowHeaderText="#{rowIndex+1}" | ||
95 | + rowHeaderPosition="both" | ||
96 | + colIndexVar="colIndex" | ||
97 | + colHeaderText="#{colIndex+1}" | ||
98 | + colHeaderPosition="both" | ||
99 | + > | ||
100 | + <coordinatesGrid:coordinatesGridItem x="#{seat.seatNr}" y="#{seat.row}" text="#{seat.row}x#{seat.seatNr}" | ||
101 | + styleClass="#{seat.enabled ? 'enabled' : 'disabled'}"/> | ||
102 | + </coordinatesGrid:coordinatesGrid> | ||
103 | + </h:panelGrid> | ||
104 | +</h:form> | ||
105 | + | ||
106 | +<div id="status"></div> | ||
107 | +<rich:insert src="/index.xhtml" highlight="xhtml" rendered="#{showSource!=false}"/> | ||
108 | + | ||
109 | +</body> | ||
110 | +</html> |
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" | ||
2 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
3 | + xmlns:f="http://java.sun.com/jsf/core" | ||
4 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
5 | + <h:panelGrid colsumns="3"> | ||
6 | + <h:outputLink value="#{facesContext.externalContext.requestContextPath}/index.jsf">Example 1</h:outputLink> | ||
7 | + </h:panelGrid> | ||
8 | +</ui:component> |
samples/dock-sample/pom.xml
0 → 100644
1 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
2 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
3 | + <parent> | ||
4 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
5 | + <artifactId>parent</artifactId> | ||
6 | + <version>3.3.4-SNAPSHOT</version> | ||
7 | + <relativePath>../pom.xml</relativePath> | ||
8 | + </parent> | ||
9 | + <modelVersion>4.0.0</modelVersion> | ||
10 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
11 | + <artifactId>dock-sample</artifactId> | ||
12 | + <packaging>war</packaging> | ||
13 | + <name>dock-sample Maven Webapp</name> | ||
14 | + <build> | ||
15 | + <finalName>${project.artifactId}</finalName> | ||
16 | + </build> | ||
17 | + <dependencies> | ||
18 | + <dependency> | ||
19 | + <groupId>org.richfaces.ui</groupId> | ||
20 | + <artifactId>richfaces-ui</artifactId> | ||
21 | + </dependency> | ||
22 | + <dependency> | ||
23 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
24 | + <artifactId>dock</artifactId> | ||
25 | + <version>${project.version}</version> | ||
26 | + </dependency> | ||
27 | + <dependency> | ||
28 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
29 | + <artifactId>notify</artifactId> | ||
30 | + <version>${project.version}</version> | ||
31 | + </dependency> | ||
32 | + <dependency> | ||
33 | + <groupId>com.uwyn</groupId> | ||
34 | + <artifactId>jhighlight</artifactId> | ||
35 | + <version>1.0</version> | ||
36 | + </dependency> | ||
37 | + </dependencies> | ||
38 | +</project> | ||
39 | + |
1 | +package org.richfaces.sandbox.dock; | ||
2 | + | ||
3 | +import javax.faces.application.FacesMessage; | ||
4 | +import javax.faces.context.FacesContext; | ||
5 | + | ||
6 | +public class Greeter { | ||
7 | + | ||
8 | + public String sayHello() { | ||
9 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_INFO, "Hello", | ||
10 | + "You've just made JSF postback. Isn't this great!")); | ||
11 | + return "hello"; | ||
12 | + } | ||
13 | + | ||
14 | + public String sayHelloAjax() { | ||
15 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_INFO, "Hello", | ||
16 | + "This is AJAX request. Isn't this even greater!")); | ||
17 | + return "helloAjax"; | ||
18 | + } | ||
19 | + | ||
20 | +} |
1 | +<?xml version="1.0"?> | ||
2 | +<!DOCTYPE faces-config PUBLIC "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" | ||
3 | + "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> | ||
4 | +<faces-config> | ||
5 | + <managed-bean> | ||
6 | + <managed-bean-name>greeter</managed-bean-name> | ||
7 | + <managed-bean-class>org.richfaces.sandbox.dock.Greeter</managed-bean-class> | ||
8 | + <managed-bean-scope>request</managed-bean-scope> | ||
9 | + </managed-bean> | ||
10 | +</faces-config> | ||
11 | + |
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
3 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
4 | + <description>Richfaces Notify Sample</description> | ||
5 | + <display-name>notify-sample</display-name> | ||
6 | + <context-param> | ||
7 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
8 | + <param-value>.xhtml</param-value> | ||
9 | + </context-param> | ||
10 | + <context-param> | ||
11 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
12 | + <param-value>2</param-value> | ||
13 | + </context-param> | ||
14 | + <context-param> | ||
15 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
16 | + <param-value>true</param-value> | ||
17 | + </context-param> | ||
18 | + <context-param> | ||
19 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
20 | + <param-value>server</param-value> | ||
21 | + </context-param> | ||
22 | + <context-param> | ||
23 | + <param-name>com.sun.faces.validateXml</param-name> | ||
24 | + <param-value>true</param-value> | ||
25 | + </context-param> | ||
26 | + <context-param> | ||
27 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
28 | + <param-value>false</param-value> | ||
29 | + </context-param> | ||
30 | + <context-param> | ||
31 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
32 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
33 | + </context-param> | ||
34 | + <context-param> | ||
35 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
36 | + <param-value>false</param-value> | ||
37 | + </context-param> | ||
38 | + <context-param> | ||
39 | + <param-name>org.richfaces.SKIN</param-name> | ||
40 | + <param-value>blueSky</param-value> | ||
41 | + </context-param> | ||
42 | + <context-param> | ||
43 | + <param-name>org.richfaces.CONTROL_SKINNING_LEVEL</param-name> | ||
44 | + <param-value>extended</param-value> | ||
45 | + </context-param> | ||
46 | + <filter> | ||
47 | + <display-name>Ajax4jsf Filter</display-name> | ||
48 | + <filter-name>ajax4jsf</filter-name> | ||
49 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
50 | + <init-param> | ||
51 | + <param-name>enable-cache</param-name> | ||
52 | + <param-value>false</param-value> | ||
53 | + </init-param> | ||
54 | + </filter> | ||
55 | + <filter-mapping> | ||
56 | + <filter-name>ajax4jsf</filter-name> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <dispatcher>FORWARD</dispatcher> | ||
59 | + <dispatcher>REQUEST</dispatcher> | ||
60 | + <dispatcher>INCLUDE</dispatcher> | ||
61 | + </filter-mapping> | ||
62 | + <servlet> | ||
63 | + <servlet-name>Faces Servlet</servlet-name> | ||
64 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
65 | + <load-on-startup>1</load-on-startup> | ||
66 | + </servlet> | ||
67 | + <servlet-mapping> | ||
68 | + <servlet-name>Faces Servlet</servlet-name> | ||
69 | + <url-pattern>*.jsf</url-pattern> | ||
70 | + </servlet-mapping> | ||
71 | + <login-config> | ||
72 | + <auth-method>BASIC</auth-method> | ||
73 | + </login-config> | ||
74 | +</web-app> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:a4j="http://richfaces.org/a4j" | ||
8 | + xmlns:rich="http://richfaces.org/rich" | ||
9 | + xmlns:dock="http://richfaces.org/sandbox/dock" | ||
10 | + xmlns:notify="http://richfaces.org/sandbox/notify" | ||
11 | + > | ||
12 | +<head> | ||
13 | + <title>Dock sample</title> | ||
14 | + <style type="text/css"> | ||
15 | + .rf-dk .jqDockLabelText { | ||
16 | + font-size: 16px; | ||
17 | + } | ||
18 | + | ||
19 | + .bottom { | ||
20 | + position: fixed; | ||
21 | + bottom: 0; | ||
22 | + width:100%; | ||
23 | + } | ||
24 | + .bottom .jqDockWrap { | ||
25 | + margin: 0 auto; | ||
26 | + } | ||
27 | + | ||
28 | + </style> | ||
29 | +</head> | ||
30 | +<body class="rich-container"> | ||
31 | +<ui:include src="menu.xhtml"/> | ||
32 | +<notify:notifyMessages ajaxRendered="true" showDetail="true"/> | ||
33 | + | ||
34 | +<p> | ||
35 | + Here we have notify displaying all messages (not only global). | ||
36 | + Severity is reflected in overriden style classes. | ||
37 | +</p> | ||
38 | +<h:form> | ||
39 | + | ||
40 | + <dock:dock> | ||
41 | + <h:outputLink title="Output link"> | ||
42 | + <h:graphicImage value="images/next.png"/> | ||
43 | + </h:outputLink> | ||
44 | + | ||
45 | + <h:commandLink title="Command link" action="#{greeter.sayHello}"> | ||
46 | + <h:graphicImage value="images/pause.png"/> | ||
47 | + </h:commandLink> | ||
48 | + | ||
49 | + <h:graphicImage value="images/play.png" title="Image without link but with a4j:support"> | ||
50 | + <a4j:support event="onclick" action="#{greeter.sayHelloAjax}" ajaxSingle="true"/> | ||
51 | + </h:graphicImage> | ||
52 | + | ||
53 | + <h:outputLink title="Link defined on link"> | ||
54 | + <h:graphicImage value="images/previous.png"/> | ||
55 | + </h:outputLink> | ||
56 | + | ||
57 | + <a title="Regular link with regular image" onclick="alert('I\'m so static')"> | ||
58 | + <img src="images/stop.png" alt=""/> | ||
59 | + </a> | ||
60 | + </dock:dock> | ||
61 | + | ||
62 | + <dock:dock align="bottom" labels="tc" styleClass="bottom"> | ||
63 | + <h:outputLink title="Output link"> | ||
64 | + <h:graphicImage value="images/next.png"/> | ||
65 | + </h:outputLink> | ||
66 | + | ||
67 | + <h:commandLink title="Command link" action="#{greeter.sayHello}"> | ||
68 | + <h:graphicImage value="images/pause.png"/> | ||
69 | + </h:commandLink> | ||
70 | + | ||
71 | + <h:graphicImage value="images/play.png" title="Image without link but with a4j:support"> | ||
72 | + <a4j:support event="onclick" action="#{greeter.sayHelloAjax}" ajaxSingle="true"/> | ||
73 | + </h:graphicImage> | ||
74 | + | ||
75 | + <h:outputLink title="Link defined on link"> | ||
76 | + <h:graphicImage value="images/previous.png"/> | ||
77 | + </h:outputLink> | ||
78 | + | ||
79 | + <a title="Regular link with regular image" onclick="alert('I\'m so static')"> | ||
80 | + <img src="images/stop.png" alt=""/> | ||
81 | + </a> | ||
82 | + </dock:dock> | ||
83 | + | ||
84 | + <dock:dock align="left" labels="mr" style="position:absolute;left:0;"> | ||
85 | + <h:outputLink title="Output link"> | ||
86 | + <h:graphicImage value="images/next.png"/> | ||
87 | + </h:outputLink> | ||
88 | + | ||
89 | + <h:commandLink title="Command link" action="#{greeter.sayHello}"> | ||
90 | + <h:graphicImage value="images/pause.png"/> | ||
91 | + </h:commandLink> | ||
92 | + | ||
93 | + <h:graphicImage value="images/play.png" title="Image without link but with a4j:support"> | ||
94 | + <a4j:support event="onclick" action="#{greeter.sayHelloAjax}" ajaxSingle="true"/> | ||
95 | + </h:graphicImage> | ||
96 | + | ||
97 | + <h:outputLink title="Link defined on link"> | ||
98 | + <h:graphicImage value="images/previous.png"/> | ||
99 | + </h:outputLink> | ||
100 | + | ||
101 | + <a title="Regular link with regular image" onclick="alert('I\'m so static')"> | ||
102 | + <img src="images/stop.png" alt=""/> | ||
103 | + </a> | ||
104 | + </dock:dock> | ||
105 | + | ||
106 | +</h:form> | ||
107 | + | ||
108 | +<rich:insert src="/dock.xhtml" highlight="xhtml"/> | ||
109 | + | ||
110 | +</body> | ||
111 | +</html> |
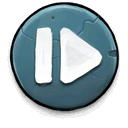
6.26 KB
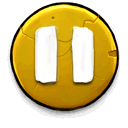
6.06 KB
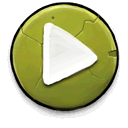
6.26 KB
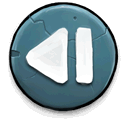
6.23 KB
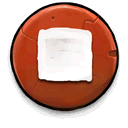
6.14 KB
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" | ||
2 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
3 | + xmlns:f="http://java.sun.com/jsf/core" | ||
4 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
5 | + <h:panelGrid columns="3"> | ||
6 | + <h:outputLink value="#{facesContext.externalContext.requestContextPath}/dock.jsf">Example 1</h:outputLink> | ||
7 | + </h:panelGrid> | ||
8 | +</ui:component> |
samples/focus-sample/pom.xml
0 → 100644
1 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
2 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
3 | + <parent> | ||
4 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
5 | + <artifactId>parent</artifactId> | ||
6 | + <version>3.3.4-SNAPSHOT</version> | ||
7 | + <relativePath>../pom.xml</relativePath> | ||
8 | + </parent> | ||
9 | + <modelVersion>4.0.0</modelVersion> | ||
10 | + <groupId>org.richfaces</groupId> | ||
11 | + <artifactId>focus-sample</artifactId> | ||
12 | + <packaging>war</packaging> | ||
13 | + <name>focus-sample Maven Webapp</name> | ||
14 | + <build> | ||
15 | + <finalName>${project.artifactId}</finalName> | ||
16 | + </build> | ||
17 | + <dependencies> | ||
18 | + <dependency> | ||
19 | + <groupId>org.richfaces.ui</groupId> | ||
20 | + <artifactId>core</artifactId> | ||
21 | + <version>${project.version}</version> | ||
22 | + </dependency> | ||
23 | + <dependency> | ||
24 | + <groupId>org.richfaces.ui</groupId> | ||
25 | + <artifactId>richfaces-ui</artifactId> | ||
26 | + </dependency> | ||
27 | + <dependency> | ||
28 | + <groupId>com.uwyn</groupId> | ||
29 | + <artifactId>jhighlight</artifactId> | ||
30 | + <version>1.0</version> | ||
31 | + </dependency> | ||
32 | + <dependency> | ||
33 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
34 | + <artifactId>focus</artifactId> | ||
35 | + <version>${project.version}</version> | ||
36 | + </dependency> | ||
37 | + </dependencies> | ||
38 | +</project> |
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" | ||
3 | + xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
4 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
5 | + <description>Richfaces Focus Sample</description> | ||
6 | + <display-name>focus-sample</display-name> | ||
7 | + <context-param> | ||
8 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
9 | + <param-value>.xhtml</param-value> | ||
10 | + </context-param> | ||
11 | + <context-param> | ||
12 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
13 | + <param-value>2</param-value> | ||
14 | + </context-param> | ||
15 | + <context-param> | ||
16 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
17 | + <param-value>true</param-value> | ||
18 | + </context-param> | ||
19 | + <context-param> | ||
20 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
21 | + <param-value>client</param-value> | ||
22 | + </context-param> | ||
23 | + <context-param> | ||
24 | + <param-name>com.sun.faces.validateXml</param-name> | ||
25 | + <param-value>true</param-value> | ||
26 | + </context-param> | ||
27 | + <context-param> | ||
28 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
29 | + <param-value>true</param-value> | ||
30 | + </context-param> | ||
31 | + <context-param> | ||
32 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
33 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
34 | + </context-param> | ||
35 | + <context-param> | ||
36 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
37 | + <param-value>false</param-value> | ||
38 | + </context-param> | ||
39 | + <filter> | ||
40 | + <display-name>Ajax4jsf Filter</display-name> | ||
41 | + <filter-name>ajax4jsf</filter-name> | ||
42 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
43 | + </filter> | ||
44 | + <filter-mapping> | ||
45 | + <filter-name>ajax4jsf</filter-name> | ||
46 | + <servlet-name>Faces Servlet</servlet-name> | ||
47 | + <dispatcher>FORWARD</dispatcher> | ||
48 | + <dispatcher>REQUEST</dispatcher> | ||
49 | + <dispatcher>INCLUDE</dispatcher> | ||
50 | + </filter-mapping> | ||
51 | + <servlet> | ||
52 | + <servlet-name>Faces Servlet</servlet-name> | ||
53 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
54 | + <load-on-startup>1</load-on-startup> | ||
55 | + </servlet> | ||
56 | + <servlet-mapping> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <url-pattern>*.jsf</url-pattern> | ||
59 | + </servlet-mapping> | ||
60 | + <login-config> | ||
61 | + <auth-method>BASIC</auth-method> | ||
62 | + </login-config> | ||
63 | +</web-app> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:focus="http://richfaces.org/sandbox/focus" | ||
8 | + xmlns:a4j="http://richfaces.org/a4j" xmlns:rich="http://richfaces.org/rich" | ||
9 | + > | ||
10 | +<head> | ||
11 | + <title>Focus sample</title> | ||
12 | +</head> | ||
13 | +<body> | ||
14 | +<ui:include src="menu.xhtml"/> | ||
15 | + | ||
16 | +<p>Focus should be placed on email: | ||
17 | + <ul> | ||
18 | + <li>username gets default priority 0</li> | ||
19 | + <li>email gets priority -1</li> | ||
20 | + </ul> | ||
21 | +</p> | ||
22 | + | ||
23 | +<h:form> | ||
24 | + <h:panelGrid columns="3"> | ||
25 | + <h:outputLabel value="Username" for="username"/> | ||
26 | + <h:inputText value="#{username}" id="username" required="true"> | ||
27 | + <focus:focus/> | ||
28 | + </h:inputText> | ||
29 | + <h:message for="username"/> | ||
30 | + | ||
31 | + <h:outputLabel value="Email" for="email"/> | ||
32 | + <h:inputText value="#{email}" id="email" required="true"/> | ||
33 | + <h:message for="email"/> | ||
34 | + </h:panelGrid> | ||
35 | + <h:commandButton value="Submit"/> | ||
36 | + <focus:focus for="email" priority="-1"/> | ||
37 | +</h:form> | ||
38 | + | ||
39 | +<rich:insert src="/focus_1.xhtml" highlight="html"/> | ||
40 | + | ||
41 | +</body> | ||
42 | +</html> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:focus="http://richfaces.org/sandbox/focus" xmlns:rich="http://richfaces.org/rich" | ||
8 | + > | ||
9 | +<head> | ||
10 | + <title>Focus sample</title> | ||
11 | +</head> | ||
12 | +<body> | ||
13 | +<ui:include src="menu.xhtml"/> | ||
14 | + | ||
15 | +<p>Focus should be placed on first radio : | ||
16 | + <ul> | ||
17 | + <li>username gets priority 9999</li> | ||
18 | + <li>email gets default priority 1</li> | ||
19 | + </ul> | ||
20 | +</p> | ||
21 | +<h:form> | ||
22 | + <h:panelGrid columns="3"> | ||
23 | + | ||
24 | + <h:outputLabel value="Username" for="username"/> | ||
25 | + <h:inputText value="#{username}" id="username" required="true"> | ||
26 | + <focus:focus priority="9999"/> | ||
27 | + </h:inputText> | ||
28 | + <h:message for="username"/> | ||
29 | + | ||
30 | + <h:outputLabel value="Option" for="option"/> | ||
31 | + <h:selectOneRadio value="#{option}" id="option" required="true"> | ||
32 | + <f:selectItem itemLabel="Option 1" itemValue="1"/> | ||
33 | + <f:selectItem itemLabel="Option 2" itemValue="2"/> | ||
34 | + <f:selectItem itemLabel="Option 3" itemValue="3"/> | ||
35 | + <focus:focus suffix=":0"/> | ||
36 | + </h:selectOneRadio> | ||
37 | + <h:message for="option"/> | ||
38 | + | ||
39 | + </h:panelGrid> | ||
40 | + <h:commandButton value="Submit"/> | ||
41 | +</h:form> | ||
42 | + | ||
43 | +<rich:insert src="/focus_2.xhtml" highlight="html"/> | ||
44 | + | ||
45 | +</body> | ||
46 | +</html> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:focus="http://richfaces.org/sandbox/focus" xmlns:rich="http://richfaces.org/rich" | ||
8 | + > | ||
9 | +<head> | ||
10 | + <title>Focus sample</title> | ||
11 | +</head> | ||
12 | +<body> | ||
13 | +<ui:include src="menu.xhtml"/> | ||
14 | + | ||
15 | +<p>Focus should be placed on username : | ||
16 | + <ul> | ||
17 | + <li>username gets default priority 0</li> | ||
18 | + <li>email gets priority 1</li> | ||
19 | + </ul> | ||
20 | +</p> | ||
21 | +<h:form> | ||
22 | + <h:panelGrid columns="3"> | ||
23 | + <h:outputLabel value="Username" for="username"/> | ||
24 | + <h:inputText value="#{username}" id="username" required="true"> | ||
25 | + <focus:focus/> | ||
26 | + </h:inputText> | ||
27 | + <h:message for="username"/> | ||
28 | + | ||
29 | + <h:outputLabel value="Email" for="email"/> | ||
30 | + <h:inputText value="#{email}" id="email" required="true"/> | ||
31 | + <h:message for="email"/> | ||
32 | + </h:panelGrid> | ||
33 | + <h:commandButton value="Submit"/> | ||
34 | + <focus:focus for="email"/> | ||
35 | +</h:form> | ||
36 | + | ||
37 | +<rich:insert src="/focus_3.xhtml" highlight="html"/> | ||
38 | + | ||
39 | +</body> | ||
40 | +</html> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" | ||
4 | + xmlns:f="http://java.sun.com/jsf/core" | ||
5 | + xmlns:h="http://java.sun.com/jsf/html" xmlns:focus="http://richfaces.org/sandbox/focus" | ||
6 | + xmlns:rich="http://richfaces.org/rich"> | ||
7 | +<head> | ||
8 | + <title>Focus sample</title> | ||
9 | +</head> | ||
10 | +<body> | ||
11 | +<ui:include src="menu.xhtml"/> | ||
12 | + | ||
13 | +<p>This example shows that focus can be put on non JSF control</p> | ||
14 | +<h:panelGrid columns="3"> | ||
15 | + <h:outputLabel value="Non JSF control" for="option"/> | ||
16 | + <input type="radio" id="option_1" name="option"/> 1 | ||
17 | + <input type="radio" id="option_2" name="option"/> 2 | ||
18 | +</h:panelGrid> | ||
19 | +<focus:focus targetClientId="option_2"/> | ||
20 | + | ||
21 | +<rich:insert src="/focus_4.xhtml" highlight="html"/> | ||
22 | + | ||
23 | +</body> | ||
24 | +</html> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:focus="http://richfaces.org/sandbox/focus" xmlns:rich="http://richfaces.org/rich" | ||
8 | + > | ||
9 | +<head> | ||
10 | + <title>Focus sample</title> | ||
11 | +</head> | ||
12 | +<body> | ||
13 | +<ui:include src="menu.xhtml"/> | ||
14 | + | ||
15 | +<p>On initial request focus should be placed on username. This is because direct parent of | ||
16 | + focus component is not UIInput. | ||
17 | + In such situation focus is put on first UIInput in parent form. | ||
18 | + Please not that focus component is not direct child of form. | ||
19 | + This sample is to ensure you that UIInput is not searched | ||
20 | + within direct parent of focus component, but within nesting form. | ||
21 | +</p> | ||
22 | +<ul> | ||
23 | + <li>username gets default priority 0</li> | ||
24 | + <li>email gets priority 1</li> | ||
25 | +</ul> | ||
26 | +<p>On form submit, when username is valid and email is invalid, focus | ||
27 | + should be placed on email. | ||
28 | +</p> | ||
29 | + | ||
30 | +<p>On form submit, when all fields are valid, focus | ||
31 | + should be placed on username. | ||
32 | +</p> | ||
33 | +<h:form> | ||
34 | + <h:panelGrid columns="3"> | ||
35 | + <h:outputLabel value="Username" for="username"/> | ||
36 | + <h:inputText value="#{username}" id="username" required="true"/> | ||
37 | + <h:message for="username"/> | ||
38 | + </h:panelGrid> | ||
39 | + <h:panelGrid columns="3"> | ||
40 | + <h:outputLabel value="Email" for="email"/> | ||
41 | + <h:inputText value="#{email}" id="email" required="true"/> | ||
42 | + <h:message for="email"/> | ||
43 | + <focus:focus/> | ||
44 | + </h:panelGrid> | ||
45 | + <h:commandButton value="Submit"/> | ||
46 | +</h:form> | ||
47 | + | ||
48 | +<rich:insert src="/focus_5.xhtml" highlight="html"/> | ||
49 | + | ||
50 | +</body> | ||
51 | +</html> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:focus="http://richfaces.org/sandbox/focus" xmlns:rich="http://richfaces.org/rich" | ||
8 | + xmlns:a4j="http://richfaces.org/a4j" | ||
9 | + > | ||
10 | +<head> | ||
11 | + <title>Focus sample</title> | ||
12 | +</head> | ||
13 | +<body> | ||
14 | +<ui:include src="menu.xhtml"/> | ||
15 | + | ||
16 | +<p> | ||
17 | + Focus is placed on control withing modal panel. Modal panel is rendered before it is shown, thus | ||
18 | + javascript that sets focus should not be invoked at that moment. | ||
19 | + To cope with that focus component has "timing" attribute which, when valued to "onJScall" | ||
20 | + allows setting focus later whenever user wishes. When "timing" is set to "onJScall" then "name" attribute | ||
21 | + must be also specified. It is name of JavaScript function that will be generated and can be called by user | ||
22 | + to set focus in any moment. | ||
23 | + In this example we put onshow="focusTheForm();" in modal panel which causes | ||
24 | + focus to be placed after the modal is visible. | ||
25 | + Notice that "name" attribute of focuse component is equal to "focusTheForm". | ||
26 | +</p> | ||
27 | +<h:form> | ||
28 | + <h:commandButton value="Show modal"> | ||
29 | + <rich:componentControl for="panel" event="onclick" operation="show" disableDefault="true"/> | ||
30 | + </h:commandButton> | ||
31 | +</h:form> | ||
32 | +<rich:modalPanel id="panel" autosized="true" onshow="focusTheForm();"> | ||
33 | + <h:form id="theForm"> | ||
34 | + <focus:focus name="focusTheForm" timing="onJScall"/> | ||
35 | + <h:panelGrid columns="3"> | ||
36 | + <h:outputLabel value="Username" for="username"/> | ||
37 | + <h:inputText value="#{username}" id="username" required="true"/> | ||
38 | + <h:message for="username"/> | ||
39 | + <h:outputLabel value="Email" for="email"/> | ||
40 | + <h:inputText value="#{email}" id="email" required="true"/> | ||
41 | + <h:message for="email"/> | ||
42 | + </h:panelGrid> | ||
43 | + <a4j:commandButton value="Submit" reRender="theForm" oncomplete="Richfaces.FocusManager.focus();"/> | ||
44 | + <h:commandButton value="Close"> | ||
45 | + <rich:componentControl for="panel" event="onclick" operation="hide" disableDefault="true"/> | ||
46 | + </h:commandButton> | ||
47 | + </h:form> | ||
48 | +</rich:modalPanel> | ||
49 | + | ||
50 | +<rich:insert src="/focus_6.xhtml" highlight="html"/> | ||
51 | + | ||
52 | +</body> | ||
53 | +</html> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" | ||
4 | + xmlns:f="http://java.sun.com/jsf/core" | ||
5 | + xmlns:h="http://java.sun.com/jsf/html" xmlns:focus="http://richfaces.org/sandbox/focus" | ||
6 | + xmlns:rich="http://richfaces.org/rich" | ||
7 | + xmlns:a4j="http://richfaces.org/a4j"> | ||
8 | +<head> | ||
9 | + <title>Focus sample</title> | ||
10 | +</head> | ||
11 | +<body> | ||
12 | +<ui:include src="menu.xhtml"/> | ||
13 | + | ||
14 | +<p> | ||
15 | + Some components like h:selectOneRadio render elements that have identifiers matching component's clientId+suffix. | ||
16 | + This might be a pain if we want to have single "focus" component inside form, but be able to focus any type of the invalid | ||
17 | + components. | ||
18 | + That's what focusModifier component is for. | ||
19 | + Some components cause even grater pain cause you cannot nest anything inside (rich:calendar). | ||
20 | + To cope with this put focusModifier inside "focusModifier" facet. </p> | ||
21 | +<h:form> | ||
22 | + <focus:focus/> | ||
23 | + <h:panelGrid columns="3"> | ||
24 | + <h:outputLabel value="Option" for="option"/> | ||
25 | + <h:selectOneRadio value="#{option}" id="option" required="true"> | ||
26 | + <f:selectItem itemLabel="Option 1" itemValue="1"/> | ||
27 | + <f:selectItem itemLabel="Option 2" itemValue="2"/> | ||
28 | + <f:selectItem itemLabel="Option 3" itemValue="3"/> | ||
29 | + <focus:focusModifier suffix=":2"/> | ||
30 | + </h:selectOneRadio> | ||
31 | + <h:message for="option"/> | ||
32 | + | ||
33 | + <h:outputLabel value="Email" for="email"/> | ||
34 | + <h:inputText value="#{email}" id="email" required="true"/> | ||
35 | + <h:message for="email"/> | ||
36 | + <h:outputLabel value="Date" for="date"/> | ||
37 | + <rich:calendar value="#{date}" id="date" enableManualInput="true" required="true"> | ||
38 | + <f:facet name="focusModifier"> | ||
39 | + <focus:focusModifier suffix="InputDate"/> | ||
40 | + </f:facet> | ||
41 | + </rich:calendar> | ||
42 | + <h:message for="date"/> | ||
43 | + </h:panelGrid> | ||
44 | + <h:commandButton value="submit"/> | ||
45 | +</h:form> | ||
46 | + | ||
47 | +<rich:insert src="/focus_7.xhtml" highlight="html"/> | ||
48 | + | ||
49 | +</body> | ||
50 | +</html> |
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" xmlns:f="http://java.sun.com/jsf/core" | ||
2 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
3 | + <div> | ||
4 | + <ul> | ||
5 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_1.jsf">Example 1</h:outputLink></li> | ||
6 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_2.jsf">Example 2</h:outputLink></li> | ||
7 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_3.jsf">Example 3</h:outputLink></li> | ||
8 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_4.jsf">Example 4</h:outputLink></li> | ||
9 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_5.jsf">Example 5</h:outputLink></li> | ||
10 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_6.jsf">Example 6</h:outputLink></li> | ||
11 | + <li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/focus_7.jsf">Example 7</h:outputLink></li> | ||
12 | + </ul> | ||
13 | + </div> | ||
14 | +</ui:component> |
samples/gmapMarker-sample/pom.xml
0 → 100644
1 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
2 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
3 | + <parent> | ||
4 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
5 | + <artifactId>parent</artifactId> | ||
6 | + <version>3.3.4-SNAPSHOT</version> | ||
7 | + <relativePath>../pom.xml</relativePath> | ||
8 | + </parent> | ||
9 | + <modelVersion>4.0.0</modelVersion> | ||
10 | + <groupId>org.richfaces</groupId> | ||
11 | + <artifactId>gmapMarker-sample</artifactId> | ||
12 | + <packaging>war</packaging> | ||
13 | + <name>gmapMarker-sample Maven Webapp</name> | ||
14 | + <build> | ||
15 | + <finalName>${project.artifactId}</finalName> | ||
16 | + </build> | ||
17 | + <dependencies> | ||
18 | + <dependency> | ||
19 | + <groupId>org.richfaces.ui</groupId> | ||
20 | + <artifactId>core</artifactId> | ||
21 | + <version>${project.version}</version> | ||
22 | + </dependency> | ||
23 | + <dependency> | ||
24 | + <groupId>org.richfaces.ui</groupId> | ||
25 | + <artifactId>richfaces-ui</artifactId> | ||
26 | + </dependency> | ||
27 | + <dependency> | ||
28 | + <groupId>com.uwyn</groupId> | ||
29 | + <artifactId>jhighlight</artifactId> | ||
30 | + <version>1.0</version> | ||
31 | + </dependency> | ||
32 | + <dependency> | ||
33 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
34 | + <artifactId>gmapMarker</artifactId> | ||
35 | + <version>${project.version}</version> | ||
36 | + </dependency> | ||
37 | + </dependencies> | ||
38 | +</project> |
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" | ||
3 | + xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
4 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
5 | + <description>Richfaces gmapMarker Sample</description> | ||
6 | + <display-name>gmapMarker-sample</display-name> | ||
7 | + <context-param> | ||
8 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
9 | + <param-value>.xhtml</param-value> | ||
10 | + </context-param> | ||
11 | + <context-param> | ||
12 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
13 | + <param-value>2</param-value> | ||
14 | + </context-param> | ||
15 | + <context-param> | ||
16 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
17 | + <param-value>true</param-value> | ||
18 | + </context-param> | ||
19 | + <context-param> | ||
20 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
21 | + <param-value>client</param-value> | ||
22 | + </context-param> | ||
23 | + <context-param> | ||
24 | + <param-name>com.sun.faces.validateXml</param-name> | ||
25 | + <param-value>true</param-value> | ||
26 | + </context-param> | ||
27 | + <context-param> | ||
28 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
29 | + <param-value>true</param-value> | ||
30 | + </context-param> | ||
31 | + <context-param> | ||
32 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
33 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
34 | + </context-param> | ||
35 | + <context-param> | ||
36 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
37 | + <param-value>false</param-value> | ||
38 | + </context-param> | ||
39 | + <filter> | ||
40 | + <display-name>Ajax4jsf Filter</display-name> | ||
41 | + <filter-name>ajax4jsf</filter-name> | ||
42 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
43 | + </filter> | ||
44 | + <filter-mapping> | ||
45 | + <filter-name>ajax4jsf</filter-name> | ||
46 | + <servlet-name>Faces Servlet</servlet-name> | ||
47 | + <dispatcher>FORWARD</dispatcher> | ||
48 | + <dispatcher>REQUEST</dispatcher> | ||
49 | + <dispatcher>INCLUDE</dispatcher> | ||
50 | + </filter-mapping> | ||
51 | + <servlet> | ||
52 | + <servlet-name>Faces Servlet</servlet-name> | ||
53 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
54 | + <load-on-startup>1</load-on-startup> | ||
55 | + </servlet> | ||
56 | + <servlet-mapping> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <url-pattern>*.jsf</url-pattern> | ||
59 | + </servlet-mapping> | ||
60 | + <login-config> | ||
61 | + <auth-method>BASIC</auth-method> | ||
62 | + </login-config> | ||
63 | + <listener> | ||
64 | + | ||
65 | + <listener-class>com.sun.faces.config.ConfigureListener</listener-class> | ||
66 | + | ||
67 | + </listener> | ||
68 | +</web-app> |
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" | ||
2 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
3 | + xmlns:f="http://java.sun.com/jsf/core" | ||
4 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
5 | + <div> | ||
6 | + <ul> | ||
7 | + <!--<li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/sample_1.jsf">Example 1</h:outputLink></li>--> | ||
8 | + </ul> | ||
9 | + </div> | ||
10 | +</ui:component> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" | ||
4 | + xmlns:f="http://java.sun.com/jsf/core" | ||
5 | + xmlns:h="http://java.sun.com/jsf/html" | ||
6 | + xmlns:sandbox="http://richfaces.org/sandbox/gmapMarker" | ||
7 | + xmlns:a4j="http://richfaces.org/a4j" | ||
8 | + xmlns:rich="http://richfaces.org/rich"> | ||
9 | +<head> | ||
10 | + <title>gmapMarker sample</title> | ||
11 | + <style type="text/css"> | ||
12 | + #calendar { | ||
13 | + float: right; | ||
14 | + width: 200px; | ||
15 | + } | ||
16 | + </style> | ||
17 | +</head> | ||
18 | + | ||
19 | +<body> | ||
20 | +<ui:include src="menu.xhtml"/> | ||
21 | + | ||
22 | + | ||
23 | +<fieldset> | ||
24 | + <legend>Add marker with JavaScript</legend> | ||
25 | + <form action="#"> | ||
26 | + Latitude <input type="text" id="lat"/> | ||
27 | + Longitude <input type="text" id="lng"/> | ||
28 | + Title <input type="text" id="title"/> | ||
29 | + Draggable <input type="checkbox" id="draggable"/> | ||
30 | + <input type="button" value="Add marker" onclick="addMarker()"/> | ||
31 | + </form> | ||
32 | + <script type="text/javascript"> | ||
33 | + function addMarker() { | ||
34 | + RichFaces.addGmapMarker('gmap', | ||
35 | + document.getElementById('lat').value, | ||
36 | + document.getElementById('lng').value, | ||
37 | + { | ||
38 | + title:document.getElementById('title').value, | ||
39 | + draggable:document.getElementById('draggable').checked | ||
40 | + }); | ||
41 | + } | ||
42 | + </script> | ||
43 | +</fieldset> | ||
44 | +<rich:gmap gmapVar="gmap" lat="50" lng="32" zoom="2" style="width:100%" | ||
45 | + gmapKey="ABQIAAAAJRoUgtOKQgPPTRmZNJcFRBQ03jzigXrzGoTeBBI15yVgqSobkRSrWVFeSVl33ncpBGX7XrKdYdUaCg"> | ||
46 | + <sandbox:gmapMarker latitude="50" longitude="32" title="Ukraine" autoPan="false" bounceGravity=".5" | ||
47 | + draggable="true" onclick="alert(marker.getTitle()+'('+latlng.lat()+';'+latlng.lng()+')')" | ||
48 | + clickable="true"/> | ||
49 | + <sandbox:gmapMarker latitude="20" longitude="52" title="Saudi Arabia - click me!" draggable="true" | ||
50 | + icon="https://issues.jboss.org/secure/attachment/12326770/richfaces_icon_64px.gif" | ||
51 | + iconWidth="32" iconHeight="32"> | ||
52 | + <f:facet name="infoWindow"> | ||
53 | + <h:panelGroup> | ||
54 | + <h:outputText value="Gubik"/><br/> | ||
55 | + <h:graphicImage value="http://in.relation.to/service/File/11358" width="300"/> | ||
56 | + </h:panelGroup> | ||
57 | + </f:facet> | ||
58 | + </sandbox:gmapMarker> | ||
59 | +</rich:gmap> | ||
60 | + | ||
61 | +<script type="text/javascript"> | ||
62 | + RichFaces.addGmapMarker('gmap', 10.0, 22.0, {title:'Toro'}); | ||
63 | +</script> | ||
64 | + | ||
65 | + | ||
66 | +<rich:insert src="/sample_1.xhtml" highlight="html"/> | ||
67 | + | ||
68 | +</body> | ||
69 | +</html> |
samples/imageSelectTool-sample/pom.xml
0 → 100644
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
3 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
4 | + <parent> | ||
5 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
6 | + <artifactId>parent</artifactId> | ||
7 | + <version>3.3.4-SNAPSHOT</version> | ||
8 | + <relativePath>../pom.xml</relativePath> | ||
9 | + </parent> | ||
10 | + <modelVersion>4.0.0</modelVersion> | ||
11 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
12 | + <artifactId>imageSelectTool-sample</artifactId> | ||
13 | + <packaging>war</packaging> | ||
14 | + <name>imageSelectTool-sample Maven Webapp</name> | ||
15 | + <build> | ||
16 | + <finalName>${project.artifactId}</finalName> | ||
17 | + </build> | ||
18 | + <dependencies> | ||
19 | + <dependency> | ||
20 | + <groupId>org.richfaces.ui</groupId> | ||
21 | + <artifactId>richfaces-ui</artifactId> | ||
22 | + </dependency> | ||
23 | + <dependency> | ||
24 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
25 | + <artifactId>imageSelectTool</artifactId> | ||
26 | + <version>${project.version}</version> | ||
27 | + </dependency> | ||
28 | + <dependency> | ||
29 | + <groupId>com.uwyn</groupId> | ||
30 | + <artifactId>jhighlight</artifactId> | ||
31 | + <version>1.0</version> | ||
32 | + </dependency> | ||
33 | + </dependencies> | ||
34 | +</project> |
samples/imageSelectTool-sample/src/main/java/org/richfaces/sandbox/imageSelectTool/Cropper.java
0 → 100644
1 | +package org.richfaces.sandbox.imageSelectTool; | ||
2 | + | ||
3 | +import java.awt.*; | ||
4 | + | ||
5 | +public class Cropper { | ||
6 | + | ||
7 | + private String srcImage = "images/sample.jpg"; | ||
8 | + private Double aspectRatio; | ||
9 | + private String backgroundColor; | ||
10 | + private Double backgroundOpacity; | ||
11 | + private Integer maxHeight; | ||
12 | + private Integer maxWidth; | ||
13 | + private Integer minHeight; | ||
14 | + private Integer minWidth; | ||
15 | + private Rectangle selection; | ||
16 | + | ||
17 | + public String getSrcImage() { | ||
18 | + return srcImage; | ||
19 | + } | ||
20 | + | ||
21 | + public void setSrcImage(String srcImage) { | ||
22 | + this.srcImage = srcImage; | ||
23 | + } | ||
24 | + | ||
25 | + public Double getAspectRatio() { | ||
26 | + return aspectRatio; | ||
27 | + } | ||
28 | + | ||
29 | + public void setAspectRatio(Double aspectRatio) { | ||
30 | + this.aspectRatio = aspectRatio; | ||
31 | + } | ||
32 | + | ||
33 | + public String getBackgroundColor() { | ||
34 | + return backgroundColor; | ||
35 | + } | ||
36 | + | ||
37 | + public void setBackgroundColor(String backgroundColor) { | ||
38 | + this.backgroundColor = backgroundColor; | ||
39 | + } | ||
40 | + | ||
41 | + public Double getBackgroundOpacity() { | ||
42 | + return backgroundOpacity; | ||
43 | + } | ||
44 | + | ||
45 | + public void setBackgroundOpacity(Double backgroundOpacity) { | ||
46 | + this.backgroundOpacity = backgroundOpacity; | ||
47 | + } | ||
48 | + | ||
49 | + public Integer getMaxHeight() { | ||
50 | + return maxHeight; | ||
51 | + } | ||
52 | + | ||
53 | + public void setMaxHeight(Integer maxHeight) { | ||
54 | + this.maxHeight = maxHeight; | ||
55 | + } | ||
56 | + | ||
57 | + public Integer getMaxWidth() { | ||
58 | + return maxWidth; | ||
59 | + } | ||
60 | + | ||
61 | + public void setMaxWidth(Integer maxWidth) { | ||
62 | + this.maxWidth = maxWidth; | ||
63 | + } | ||
64 | + | ||
65 | + public Integer getMinHeight() { | ||
66 | + return minHeight; | ||
67 | + } | ||
68 | + | ||
69 | + public void setMinHeight(Integer minHeight) { | ||
70 | + this.minHeight = minHeight; | ||
71 | + } | ||
72 | + | ||
73 | + public Integer getMinWidth() { | ||
74 | + return minWidth; | ||
75 | + } | ||
76 | + | ||
77 | + public void setMinWidth(Integer minWidth) { | ||
78 | + this.minWidth = minWidth; | ||
79 | + } | ||
80 | + | ||
81 | + public Rectangle getSelection() { | ||
82 | + return selection; | ||
83 | + } | ||
84 | + | ||
85 | + public void setSelection(Rectangle selection) { | ||
86 | + this.selection = selection; | ||
87 | + } | ||
88 | +} |
1 | +<?xml version="1.0"?> | ||
2 | +<!DOCTYPE faces-config PUBLIC "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" | ||
3 | + "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> | ||
4 | +<faces-config> | ||
5 | + <managed-bean> | ||
6 | + <managed-bean-name>cropper</managed-bean-name> | ||
7 | + <managed-bean-class>org.richfaces.sandbox.imageSelectTool.Cropper</managed-bean-class> | ||
8 | + <managed-bean-scope>session</managed-bean-scope> | ||
9 | + </managed-bean> | ||
10 | +</faces-config> | ||
11 | + |
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
3 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
4 | + <description>Richfaces imageSelectTool Sample</description> | ||
5 | + <display-name>imageSelectTool-sample</display-name> | ||
6 | + <context-param> | ||
7 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
8 | + <param-value>.xhtml</param-value> | ||
9 | + </context-param> | ||
10 | + <context-param> | ||
11 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
12 | + <param-value>2</param-value> | ||
13 | + </context-param> | ||
14 | + <context-param> | ||
15 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
16 | + <param-value>true</param-value> | ||
17 | + </context-param> | ||
18 | + <context-param> | ||
19 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
20 | + <param-value>server</param-value> | ||
21 | + </context-param> | ||
22 | + <context-param> | ||
23 | + <param-name>com.sun.faces.validateXml</param-name> | ||
24 | + <param-value>true</param-value> | ||
25 | + </context-param> | ||
26 | + <context-param> | ||
27 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
28 | + <param-value>false</param-value> | ||
29 | + </context-param> | ||
30 | + <context-param> | ||
31 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
32 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
33 | + </context-param> | ||
34 | + <context-param> | ||
35 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
36 | + <param-value>false</param-value> | ||
37 | + </context-param> | ||
38 | + <context-param> | ||
39 | + <param-name>org.richfaces.SKIN</param-name> | ||
40 | + <param-value>blueSky</param-value> | ||
41 | + </context-param> | ||
42 | + <context-param> | ||
43 | + <param-name>org.richfaces.CONTROL_SKINNING_LEVEL</param-name> | ||
44 | + <param-value>extended</param-value> | ||
45 | + </context-param> | ||
46 | + <filter> | ||
47 | + <display-name>Ajax4jsf Filter</display-name> | ||
48 | + <filter-name>ajax4jsf</filter-name> | ||
49 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
50 | + <init-param> | ||
51 | + <param-name>enable-cache</param-name> | ||
52 | + <param-value>false</param-value> | ||
53 | + </init-param> | ||
54 | + </filter> | ||
55 | + <filter-mapping> | ||
56 | + <filter-name>ajax4jsf</filter-name> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <dispatcher>FORWARD</dispatcher> | ||
59 | + <dispatcher>REQUEST</dispatcher> | ||
60 | + <dispatcher>INCLUDE</dispatcher> | ||
61 | + </filter-mapping> | ||
62 | + <servlet> | ||
63 | + <servlet-name>Faces Servlet</servlet-name> | ||
64 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
65 | + <load-on-startup>1</load-on-startup> | ||
66 | + </servlet> | ||
67 | + <servlet-mapping> | ||
68 | + <servlet-name>Faces Servlet</servlet-name> | ||
69 | + <url-pattern>*.jsf</url-pattern> | ||
70 | + </servlet-mapping> | ||
71 | + <login-config> | ||
72 | + <auth-method>BASIC</auth-method> | ||
73 | + </login-config> | ||
74 | +</web-app> |
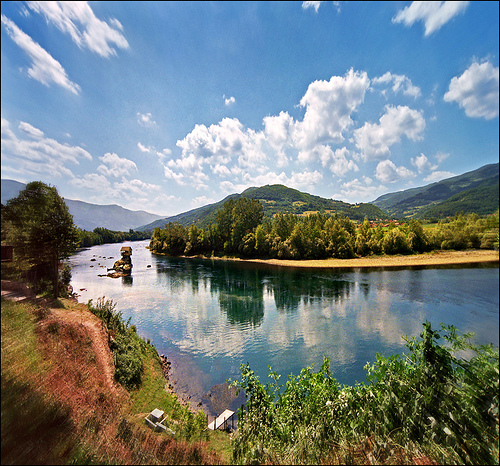
224 KB
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" | ||
4 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
5 | + xmlns:f="http://java.sun.com/jsf/core" | ||
6 | + xmlns:h="http://java.sun.com/jsf/html" | ||
7 | + xmlns:a4j="http://richfaces.org/a4j" | ||
8 | + xmlns:rich="http://richfaces.org/rich" | ||
9 | + xmlns:imageSelectTool="http://richfaces.org/sandbox/imageSelectTool" | ||
10 | + > | ||
11 | +<head> | ||
12 | + <title>ImageSelectTool sample</title> | ||
13 | + <style type="text/css"> | ||
14 | + input.short { | ||
15 | + width: 30px; | ||
16 | + } | ||
17 | + </style> | ||
18 | +</head> | ||
19 | +<body class="rich-container"> | ||
20 | +<ui:include src="menu.xhtml"/> | ||
21 | + | ||
22 | +<p> | ||
23 | + | ||
24 | +</p> | ||
25 | +<rich:messages ajaxRendered="true"/> | ||
26 | +<h:panelGrid columns="3"> | ||
27 | + <h:panelGroup> | ||
28 | + <h:form> | ||
29 | + <h:panelGrid columns="2"> | ||
30 | + <h:outputLabel value="srcImage" for="srcImage"/> | ||
31 | + <h:inputText value="#{cropper.srcImage}" id="srcImage"/> | ||
32 | + <h:outputLabel value="minWidth" for="minWidth"/> | ||
33 | + <h:inputText value="#{cropper.minWidth}" id="minWidth"/> | ||
34 | + <h:outputLabel value="minHeight" for="minHeight"/> | ||
35 | + <h:inputText value="#{cropper.minHeight}" id="minHeight"/> | ||
36 | + <h:outputLabel value="maxWidth" for="maxWidth"/> | ||
37 | + <h:inputText value="#{cropper.maxWidth}" id="maxWidth"/> | ||
38 | + <h:outputLabel value="maxHeight" for="maxHeight"/> | ||
39 | + <h:inputText value="#{cropper.maxHeight}" id="maxHeight"/> | ||
40 | + <h:outputLabel value="backgroundColor" for="backgroundColor"/> | ||
41 | + <h:inputText value="#{cropper.backgroundColor}" id="backgroundColor"/> | ||
42 | + <h:outputLabel value="backgroundOpacity" for="backgroundOpacity"/> | ||
43 | + <h:inputText value="#{cropper.backgroundOpacity}" id="backgroundOpacity"/> | ||
44 | + <h:outputLabel value="aspectRatio" for="aspectRatio"/> | ||
45 | + <h:inputText value="#{cropper.aspectRatio}" id="aspectRatio"/> | ||
46 | + </h:panelGrid> | ||
47 | + <h:commandButton value="Submit"/> | ||
48 | + </h:form> | ||
49 | + | ||
50 | + <div id="coords"/> | ||
51 | + <input type="text" id="x" class="short"/> | ||
52 | + <input type="text" id="y" class="short"/> | ||
53 | + <input type="text" id="width" class="short"/> | ||
54 | + <input type="text" id="height" class="short"/> | ||
55 | + <input type="button" id="setSelection" value="Set selection"/> | ||
56 | + <input type="button" id="animateTo" value="Animate to"/> | ||
57 | + | ||
58 | + <h:form> | ||
59 | + | ||
60 | + </h:form> | ||
61 | + </h:panelGroup> | ||
62 | + | ||
63 | + | ||
64 | + <h:graphicImage value="#{cropper.srcImage}" id="image"/> | ||
65 | + | ||
66 | + <rich:panel id="result"> | ||
67 | + #{cropper.selection} | ||
68 | + </rich:panel> | ||
69 | +</h:panelGrid> | ||
70 | +<script type="text/javascript"> | ||
71 | + function updateInputs(event) { | ||
72 | + jQuery('#coords').html('selected:' + event.x + ';' + event.y + ';' + event.width + ';' + event.height); | ||
73 | + jQuery('#x').val(event.x); | ||
74 | + jQuery('#y').val(event.y); | ||
75 | + jQuery('#width').val(event.width); | ||
76 | + jQuery('#height').val(event.height); | ||
77 | + } | ||
78 | + jQuery('#setSelection').click(function() { | ||
79 | + selector.setSelection( | ||
80 | + parseInt(jQuery('#x').val()), | ||
81 | + parseInt(jQuery('#y').val()), | ||
82 | + parseInt(jQuery('#width').val()), | ||
83 | + parseInt(jQuery('#height').val()) | ||
84 | + ); | ||
85 | + }); | ||
86 | + jQuery('#animateTo').click(function() { | ||
87 | + selector.animateTo( | ||
88 | + parseInt(jQuery('#x').val()), | ||
89 | + parseInt(jQuery('#y').val()), | ||
90 | + parseInt(jQuery('#width').val()), | ||
91 | + parseInt(jQuery('#height').val()) | ||
92 | + ); | ||
93 | + }); | ||
94 | +</script> | ||
95 | +<h:form> | ||
96 | + <imageSelectTool:imageSelectTool id="selector" | ||
97 | + for="image" | ||
98 | + var="selector" | ||
99 | + value="#{cropper.selection}" | ||
100 | + aspectRatio="#{cropper.aspectRatio}" | ||
101 | + backgroundColor="#{cropper.backgroundColor}" | ||
102 | + backgroundOpacity="#{cropper.backgroundOpacity}" | ||
103 | + maxHeight="#{cropper.maxHeight}" | ||
104 | + maxWidth="#{cropper.maxWidth}" | ||
105 | + minWidth="#{cropper.minWidth}" | ||
106 | + minHeight="#{cropper.minHeight}" | ||
107 | + onchange="updateInputs(event);" | ||
108 | + onselect="updateInputs(event);reRenderResult()"/> | ||
109 | + <a4j:jsFunction name="reRenderResult" reRender="result"/> | ||
110 | + <a4j:commandButton value="ReRender image" reRender="image"/> | ||
111 | + <a4j:commandButton value="ReRender imageSelectTool" reRender="selector"/> | ||
112 | + <a4j:commandButton value="ReRender both" reRender="selector,image"/> | ||
113 | +</h:form> | ||
114 | +<rich:insert src="/index.xhtml" highlight="xhtml" rendered="#{showSource!=false}"/> | ||
115 | + | ||
116 | +</body> | ||
117 | +</html> |
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" | ||
2 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
3 | + xmlns:f="http://java.sun.com/jsf/core" | ||
4 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
5 | + <h:panelGrid columns="3"> | ||
6 | + <h:outputLink value="#{facesContext.externalContext.requestContextPath}/index.jsf">Example 1</h:outputLink> | ||
7 | + </h:panelGrid> | ||
8 | +</ui:component> |
samples/jQueryPlugin-sample/pom.xml
0 → 100644
1 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
2 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
3 | + <parent> | ||
4 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
5 | + <artifactId>parent</artifactId> | ||
6 | + <version>3.3.4-SNAPSHOT</version> | ||
7 | + <relativePath>../pom.xml</relativePath> | ||
8 | + </parent> | ||
9 | + <modelVersion>4.0.0</modelVersion> | ||
10 | + <groupId>org.richfaces</groupId> | ||
11 | + <artifactId>jQueryPlugin-sample</artifactId> | ||
12 | + <packaging>war</packaging> | ||
13 | + <name>jQueryPlugin-sample Maven Webapp</name> | ||
14 | + <build> | ||
15 | + <finalName>${project.artifactId}</finalName> | ||
16 | + </build> | ||
17 | + <dependencies> | ||
18 | + <dependency> | ||
19 | + <groupId>org.richfaces.ui</groupId> | ||
20 | + <artifactId>core</artifactId> | ||
21 | + <version>${project.version}</version> | ||
22 | + </dependency> | ||
23 | + <dependency> | ||
24 | + <groupId>org.richfaces.ui</groupId> | ||
25 | + <artifactId>richfaces-ui</artifactId> | ||
26 | + </dependency> | ||
27 | + <dependency> | ||
28 | + <groupId>com.uwyn</groupId> | ||
29 | + <artifactId>jhighlight</artifactId> | ||
30 | + <version>1.0</version> | ||
31 | + </dependency> | ||
32 | + <dependency> | ||
33 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
34 | + <artifactId>jQueryPlugin</artifactId> | ||
35 | + <version>${project.version}</version> | ||
36 | + </dependency> | ||
37 | + </dependencies> | ||
38 | +</project> |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<f:template xmlns:f='http:/jsf.exadel.com/template' | ||
3 | +xmlns:u='http:/jsf.exadel.com/template/util' | ||
4 | +xmlns="http://www.w3.org/1999/xhtml" > | ||
5 | + | ||
6 | + <f:verbatim> | ||
7 | +/* Notice----------------------------------*/ | ||
8 | +.rf-ny { | ||
9 | + top: 18px; | ||
10 | + right: 18px; | ||
11 | + position: absolute; | ||
12 | + height: auto; | ||
13 | + /* Ensure that the notices are on top of everything else. */ | ||
14 | + z-index: 9999; | ||
15 | +} | ||
16 | + </f:verbatim> | ||
17 | + <u:selector name=".rf-ny-info"> | ||
18 | + <u:style name="color" skin="generalTextColor"/> | ||
19 | + </u:selector> | ||
20 | + <f:verbatim> | ||
21 | +.rf-ny-warn { | ||
22 | + color:orange; | ||
23 | +} | ||
24 | +.rf-ny-error { | ||
25 | + color:red; | ||
26 | +} | ||
27 | +.rf-ny-fatal { | ||
28 | + color:red; | ||
29 | + font-weight:bold; | ||
30 | +} | ||
31 | +/* This hides position: fixed from IE6, which doesn't understand it. */ | ||
32 | +html > body .rf-ny { | ||
33 | + position: fixed; | ||
34 | +} | ||
35 | + </f:verbatim> | ||
36 | + <u:selector name=".rf-ny .rf-ny-sh"> | ||
37 | + <u:style name="margin" value="0"/> | ||
38 | + <u:style name="padding" value="8px"/> | ||
39 | + <u:style name="background-color" skin="headerBackgroundColor"/> | ||
40 | + <u:style name="color" skin="headerTextColor"/> | ||
41 | + <u:style name="opcity" value=".3"/> | ||
42 | + <u:style name="-moz-border-radius" value="8px"/> | ||
43 | + <u:style name="-webkit-border-radius" value="8px"/> | ||
44 | + <u:style name="border-radius" value="8px"/> | ||
45 | + <u:style name="position" value="absolute"/> | ||
46 | + <u:style name="z-index" value="-1"/> | ||
47 | + <u:style name="top" value=".1em"/> | ||
48 | + <u:style name="left" value=".1em"/> | ||
49 | + <u:style name="bottom" value="-.2em"/> | ||
50 | + <u:style name="right" value="-.2em"/> | ||
51 | + </u:selector> | ||
52 | + <u:selector name=".rf-ny-co"> | ||
53 | + <u:style name="height" value="100%"/> | ||
54 | + <u:style name="padding" value=".8em"/> | ||
55 | + <u:style name="border-width" value="1px"/> | ||
56 | + <u:style name="border-style" value="solid"/> | ||
57 | + <u:style name="border-color" value="panelBorderColor"/> | ||
58 | + <u:style name="background-color" skin="generalBackgroundColor"/> | ||
59 | + <u:style name="color" skin="panelTextColor"/> | ||
60 | + <u:style name="-moz-border-radius" value="4px"/> | ||
61 | + <u:style name="-webkit-border-radius" value="4px"/> | ||
62 | + <u:style name="border-radius" value="4px"/> | ||
63 | + </u:selector> | ||
64 | + <f:verbatim> | ||
65 | +.rf-ny-co-hover { | ||
66 | + background:red; | ||
67 | +} | ||
68 | +.rf-ny-cl { | ||
69 | + float: right; | ||
70 | + margin-left: .2em; | ||
71 | +} | ||
72 | + </f:verbatim> | ||
73 | + <u:selector name=".rf-ny-cl-ic"> | ||
74 | + <u:style name="display" value="block"/> | ||
75 | + <u:style name="width" value="11px"/> | ||
76 | + <u:style name="height" value="11px"/> | ||
77 | + <u:style name="background-image"> | ||
78 | + <f:resource f:key="org.richfaces.renderkit.html.images.CancelControlIcon"/> | ||
79 | + </u:style> | ||
80 | + </u:selector> | ||
81 | + <f:verbatim> | ||
82 | +.rf-ny-ti { | ||
83 | + display: block; | ||
84 | + font-size: 1.2em; | ||
85 | + font-weight: bold; | ||
86 | + margin-bottom: .4em; | ||
87 | +} | ||
88 | +.rf-ny-te { | ||
89 | + display: block; | ||
90 | +} | ||
91 | +.rf-ny-ic { | ||
92 | + display: none; | ||
93 | + float: left; | ||
94 | + margin-right: .2em; | ||
95 | + width:32px; | ||
96 | + height:32px; | ||
97 | +} | ||
98 | +/* History Pulldown----------------------------------*/ | ||
99 | + </f:verbatim> | ||
100 | + <u:selector name=".rf-ny-hc"> | ||
101 | + <u:style name="background-color" skin="headerBackgroundColor"/> | ||
102 | + <u:style name="border-color" skin="headerBackgroundColor"/> | ||
103 | + <u:style name="font-size" skin="headerSizeFont"/> | ||
104 | + <u:style name="color" skin="headerTextColor"/> | ||
105 | + <u:style name="font-family" skin="headerFamilyFont"/> | ||
106 | + <u:style name="background-image"> | ||
107 | + <f:resource f:key="org.richfaces.renderkit.html.GradientA"/> | ||
108 | + </u:style> | ||
109 | + <u:style name="font-weight" value="normal"/> | ||
110 | + <u:style name="color" skin="panelTextColor"/> | ||
111 | + <u:style name="-moz-border-radius-bottomleft" value="4px"/> | ||
112 | + <u:style name="-webkit-border-bottom-left-radius" value="4px"/> | ||
113 | + <u:style name="border-bottom-left-radius" value="4px"/> | ||
114 | + <u:style name="-moz-border-radius-bottomright" value="4px"/> | ||
115 | + <u:style name="-webkit-border-bottom-right-radius" value="4px"/> | ||
116 | + <u:style name="border-bottom-right-radius" value="4px"/> | ||
117 | + <u:style name="position" value="absolute"/> | ||
118 | + <u:style name="top" value="0"/> | ||
119 | + <u:style name="right" value="18px"/> | ||
120 | + <u:style name="width" value="70px"/> | ||
121 | + <u:style name="z-index" value="10000"/> | ||
122 | + </u:selector> | ||
123 | + <f:verbatim> | ||
124 | +.rf-ny-hc .rf-ny-hh { | ||
125 | + padding: 2px; | ||
126 | +} | ||
127 | +.rf-ny-hc button { | ||
128 | + cursor: pointer; | ||
129 | + display: block; | ||
130 | + width: 100%; | ||
131 | +} | ||
132 | +.rc-ny-ha { | ||
133 | + -moz-border-radius: 4px; -webkit-border-radius: 4px; border-radius: 4px; | ||
134 | +} | ||
135 | + </f:verbatim> | ||
136 | + <u:selector name=".rf-ny-hc .rf-ny-hp"> | ||
137 | + <u:style name="display" value="block"/> | ||
138 | + <u:style name="margin" value="0 auto"/> | ||
139 | + <u:style name="width" value="16px"/> | ||
140 | + <u:style name="height" value="16px"/> | ||
141 | + <u:style name="background-color" skin="panelTextColor"/> | ||
142 | + <u:style name="background-image"> | ||
143 | + <f:resource f:key="/org/richfaces/renderkit/html/images/triangleIconDown.png"/> | ||
144 | + </u:style> | ||
145 | + </u:selector> | ||
146 | + <u:selector name=".rc-ny-hl"> | ||
147 | + <u:style name="border-color" skin="panelBorderColor"/> | ||
148 | + <u:style name="background-color" skin="generalBackgroundColor"/> | ||
149 | + <u:style name="font-weight" value="normal"/> | ||
150 | + <u:style name="color" skin="panelTextColor"/> | ||
151 | + <u:style name="-moz-border-radius" value="4px"/> | ||
152 | + <u:style name="-webkit-border-radius" value="4px"/> | ||
153 | + <u:style name="border-radius" value="4px"/> | ||
154 | + </u:selector> | ||
155 | + <u:selector name=".rf-ny-info .rf-ny-ic"> | ||
156 | + <u:style name="display" value="block"/> | ||
157 | + <u:style name="background-image"> | ||
158 | + <f:resource f:key="/org/richfaces/renderkit/html/images/info.png"/> | ||
159 | + </u:style> | ||
160 | + </u:selector> | ||
161 | + <u:selector name=".rf-ny-warn .rf-ny-ic"> | ||
162 | + <u:style name="display" value="block"/> | ||
163 | + <u:style name="background-image"> | ||
164 | + <f:resource f:key="/org/richfaces/renderkit/html/images/warn.png"/> | ||
165 | + </u:style> | ||
166 | + </u:selector> | ||
167 | + <u:selector name=".rf-ny-error .rf-ny-ic"> | ||
168 | + <u:style name="display" value="block"/> | ||
169 | + <u:style name="background-image"> | ||
170 | + <f:resource f:key="/org/richfaces/renderkit/html/images/error.png"/> | ||
171 | + </u:style> | ||
172 | + </u:selector> | ||
173 | + <u:selector name=".rf-ny-fatal .rf-ny-ic"> | ||
174 | + <u:style name="display" value="block"/> | ||
175 | + <u:style name="background-image"> | ||
176 | + <f:resource f:key="/org/richfaces/renderkit/html/images/fatal.png"/> | ||
177 | + </u:style> | ||
178 | + </u:selector> | ||
179 | +</f:template> |
samples/jQueryPlugin-sample/src/main/resources/org/richfaces/renderkit/html/images/error.png
0 → 100644

1.97 KB
samples/jQueryPlugin-sample/src/main/resources/org/richfaces/renderkit/html/images/fatal.png
0 → 100644

2.41 KB
samples/jQueryPlugin-sample/src/main/resources/org/richfaces/renderkit/html/images/info.png
0 → 100644

4.49 KB

74 Bytes
samples/jQueryPlugin-sample/src/main/resources/org/richfaces/renderkit/html/images/warn.png
0 → 100644

1.38 KB
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" | ||
3 | + xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
4 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
5 | + <description>Richfaces jQueryPlugin Sample</description> | ||
6 | + <display-name>jQueryPlugin-sample</display-name> | ||
7 | + <context-param> | ||
8 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
9 | + <param-value>.xhtml</param-value> | ||
10 | + </context-param> | ||
11 | + <context-param> | ||
12 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
13 | + <param-value>2</param-value> | ||
14 | + </context-param> | ||
15 | + <context-param> | ||
16 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
17 | + <param-value>true</param-value> | ||
18 | + </context-param> | ||
19 | + <context-param> | ||
20 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
21 | + <param-value>client</param-value> | ||
22 | + </context-param> | ||
23 | + <context-param> | ||
24 | + <param-name>com.sun.faces.validateXml</param-name> | ||
25 | + <param-value>true</param-value> | ||
26 | + </context-param> | ||
27 | + <context-param> | ||
28 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
29 | + <param-value>true</param-value> | ||
30 | + </context-param> | ||
31 | + <context-param> | ||
32 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
33 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
34 | + </context-param> | ||
35 | + <context-param> | ||
36 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
37 | + <param-value>false</param-value> | ||
38 | + </context-param> | ||
39 | + <filter> | ||
40 | + <display-name>Ajax4jsf Filter</display-name> | ||
41 | + <filter-name>ajax4jsf</filter-name> | ||
42 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
43 | + </filter> | ||
44 | + <filter-mapping> | ||
45 | + <filter-name>ajax4jsf</filter-name> | ||
46 | + <servlet-name>Faces Servlet</servlet-name> | ||
47 | + <dispatcher>FORWARD</dispatcher> | ||
48 | + <dispatcher>REQUEST</dispatcher> | ||
49 | + <dispatcher>INCLUDE</dispatcher> | ||
50 | + </filter-mapping> | ||
51 | + <servlet> | ||
52 | + <servlet-name>Faces Servlet</servlet-name> | ||
53 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
54 | + <load-on-startup>1</load-on-startup> | ||
55 | + </servlet> | ||
56 | + <servlet-mapping> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <url-pattern>*.jsf</url-pattern> | ||
59 | + </servlet-mapping> | ||
60 | + <login-config> | ||
61 | + <auth-method>BASIC</auth-method> | ||
62 | + </login-config> | ||
63 | + <listener> | ||
64 | + | ||
65 | + <listener-class>com.sun.faces.config.ConfigureListener</listener-class> | ||
66 | + | ||
67 | + </listener> | ||
68 | +</web-app> |
This diff could not be displayed because it is too large.
1 | +/* | ||
2 | + * jQuery Pines Notify (pnotify) Plugin 1.0.0 | ||
3 | + * | ||
4 | + * Copyright (c) 2009 Hunter Perrin | ||
5 | + * | ||
6 | + * Licensed (along with all of Pines) under the GNU Affero GPL: | ||
7 | + * http://www.gnu.org/licenses/agpl.html | ||
8 | + */ | ||
9 | + | ||
10 | +(function($) { | ||
11 | + var history_handle_top, timer; | ||
12 | + var body; | ||
13 | + var jwindow; | ||
14 | + | ||
15 | + function $14(element, options) { | ||
16 | + var attrFn = { | ||
17 | + val: true, | ||
18 | + css: true, | ||
19 | + html: true, | ||
20 | + text: true, | ||
21 | + data: true, | ||
22 | + width: true, | ||
23 | + height: true, | ||
24 | + offset: true | ||
25 | + }; | ||
26 | + var o = jQuery(element); | ||
27 | + for (var key in options) { | ||
28 | + if (jQuery.isFunction(options[key])) { | ||
29 | + o.bind(key, options[key]); | ||
30 | + } else if (key in attrFn) { | ||
31 | + o[key](options[key]); | ||
32 | + } else { | ||
33 | + o.attr(key, options[key]); | ||
34 | + } | ||
35 | + } | ||
36 | + return o; | ||
37 | + } | ||
38 | + | ||
39 | + $.extend({ | ||
40 | + pnotify_remove_all: function () { | ||
41 | + var body_data = body.data("pnotify"); | ||
42 | + /* POA: Added null-check */ | ||
43 | + if (body_data && body_data.length) { | ||
44 | + $.each(body_data, function() { | ||
45 | + if (this.pnotify_remove) | ||
46 | + this.pnotify_remove(); | ||
47 | + }); | ||
48 | + } | ||
49 | + }, | ||
50 | + pnotify_position_all: function () { | ||
51 | + if (timer) | ||
52 | + clearTimeout(timer); | ||
53 | + timer = null; | ||
54 | + var body_data = body.data("pnotify"); | ||
55 | + if (!body_data || !body_data.length) | ||
56 | + return; | ||
57 | + $.each(body_data, function() { | ||
58 | + var s = this.opts.pnotify_stack; | ||
59 | + if (!s) return; | ||
60 | + if (!s.nextpos1) | ||
61 | + s.nextpos1 = s.firstpos1; | ||
62 | + if (!s.nextpos2) | ||
63 | + s.nextpos2 = s.firstpos2; | ||
64 | + if (!s.addpos2) | ||
65 | + s.addpos2 = 0; | ||
66 | + if (this.css("display") != "none") { | ||
67 | + var curpos1, curpos2; | ||
68 | + var animate = {}; | ||
69 | + // Calculate the current pos1 value. | ||
70 | + var csspos1; | ||
71 | + switch (s.dir1) { | ||
72 | + case "down": | ||
73 | + csspos1 = "top"; | ||
74 | + break; | ||
75 | + case "up": | ||
76 | + csspos1 = "bottom"; | ||
77 | + break; | ||
78 | + case "left": | ||
79 | + csspos1 = "right"; | ||
80 | + break; | ||
81 | + case "right": | ||
82 | + csspos1 = "left"; | ||
83 | + break; | ||
84 | + } | ||
85 | + curpos1 = parseInt(this.css(csspos1)); | ||
86 | + if (isNaN(curpos1)) | ||
87 | + curpos1 = 0; | ||
88 | + // Remember the first pos1, so the first visible notice goes there. | ||
89 | + if (typeof s.firstpos1 == "undefined") { | ||
90 | + s.firstpos1 = curpos1; | ||
91 | + s.nextpos1 = s.firstpos1; | ||
92 | + } | ||
93 | + // Calculate the current pos2 value. | ||
94 | + var csspos2; | ||
95 | + switch (s.dir2) { | ||
96 | + case "down": | ||
97 | + csspos2 = "top"; | ||
98 | + break; | ||
99 | + case "up": | ||
100 | + csspos2 = "bottom"; | ||
101 | + break; | ||
102 | + case "left": | ||
103 | + csspos2 = "right"; | ||
104 | + break; | ||
105 | + case "right": | ||
106 | + csspos2 = "left"; | ||
107 | + break; | ||
108 | + } | ||
109 | + curpos2 = parseInt(this.css(csspos2)); | ||
110 | + if (isNaN(curpos2)) | ||
111 | + curpos2 = 0; | ||
112 | + // Remember the first pos2, so the first visible notice goes there. | ||
113 | + if (typeof s.firstpos2 == "undefined") { | ||
114 | + s.firstpos2 = curpos2; | ||
115 | + s.nextpos2 = s.firstpos2; | ||
116 | + } | ||
117 | + // Check that it's not beyond the viewport edge. | ||
118 | + if ((s.dir1 == "down" && s.nextpos1 + this.height() > jwindow.height()) || | ||
119 | + (s.dir1 == "up" && s.nextpos1 + this.height() > jwindow.height()) || | ||
120 | + (s.dir1 == "left" && s.nextpos1 + this.width() > jwindow.width()) || | ||
121 | + (s.dir1 == "right" && s.nextpos1 + this.width() > jwindow.width())) { | ||
122 | + // If it is, it needs to go back to the first pos1, and over on pos2. | ||
123 | + s.nextpos1 = s.firstpos1; | ||
124 | + s.nextpos2 += s.addpos2 + 10; | ||
125 | + s.addpos2 = 0; | ||
126 | + } | ||
127 | + // Animate if we're moving on dir2. | ||
128 | + if (s.animation && s.nextpos2 < curpos2) { | ||
129 | + switch (s.dir2) { | ||
130 | + case "down": | ||
131 | + animate.top = s.nextpos2 + "px"; | ||
132 | + break; | ||
133 | + case "up": | ||
134 | + animate.bottom = s.nextpos2 + "px"; | ||
135 | + break; | ||
136 | + case "left": | ||
137 | + animate.right = s.nextpos2 + "px"; | ||
138 | + break; | ||
139 | + case "right": | ||
140 | + animate.left = s.nextpos2 + "px"; | ||
141 | + break; | ||
142 | + } | ||
143 | + } else | ||
144 | + this.css(csspos2, s.nextpos2 + "px"); | ||
145 | + // Keep track of the widest/tallest notice in the column/row, so we can push the next column/row. | ||
146 | + switch (s.dir2) { | ||
147 | + case "down": | ||
148 | + case "up": | ||
149 | + if (this.outerHeight(true) > s.addpos2) | ||
150 | + s.addpos2 = this.height(); | ||
151 | + break; | ||
152 | + case "left": | ||
153 | + case "right": | ||
154 | + if (this.outerWidth(true) > s.addpos2) | ||
155 | + s.addpos2 = this.width(); | ||
156 | + break; | ||
157 | + } | ||
158 | + // Move the notice on dir1. | ||
159 | + if (s.nextpos1) { | ||
160 | + // Animate if we're moving toward the first pos. | ||
161 | + if (s.animation && (curpos1 > s.nextpos1 || animate.top || animate.bottom || animate.right || animate.left)) { | ||
162 | + switch (s.dir1) { | ||
163 | + case "down": | ||
164 | + animate.top = s.nextpos1 + "px"; | ||
165 | + break; | ||
166 | + case "up": | ||
167 | + animate.bottom = s.nextpos1 + "px"; | ||
168 | + break; | ||
169 | + case "left": | ||
170 | + animate.right = s.nextpos1 + "px"; | ||
171 | + break; | ||
172 | + case "right": | ||
173 | + animate.left = s.nextpos1 + "px"; | ||
174 | + break; | ||
175 | + } | ||
176 | + } else | ||
177 | + this.css(csspos1, s.nextpos1 + "px"); | ||
178 | + } | ||
179 | + if (animate.top || animate.bottom || animate.right || animate.left) | ||
180 | + this.animate(animate, {duration: 500, queue: false}); | ||
181 | + // Calculate the next dir1 position. | ||
182 | + switch (s.dir1) { | ||
183 | + case "down": | ||
184 | + case "up": | ||
185 | + s.nextpos1 += this.height() + 10; | ||
186 | + break; | ||
187 | + case "left": | ||
188 | + case "right": | ||
189 | + s.nextpos1 += this.width() + 10; | ||
190 | + break; | ||
191 | + } | ||
192 | + } | ||
193 | + }); | ||
194 | + // Reset the next position data. | ||
195 | + $.each(body_data, function() { | ||
196 | + var s = this.opts.pnotify_stack; | ||
197 | + if (!s) return; | ||
198 | + s.nextpos1 = s.firstpos1; | ||
199 | + s.nextpos2 = s.firstpos2; | ||
200 | + s.addpos2 = 0; | ||
201 | + s.animation = true; | ||
202 | + }); | ||
203 | + }, | ||
204 | + pnotify: function(options) { | ||
205 | + if (!body) | ||
206 | + body = $("body"); | ||
207 | + if (!jwindow) | ||
208 | + jwindow = $(window); | ||
209 | + | ||
210 | + var animating; | ||
211 | + | ||
212 | + // Build main options. | ||
213 | + var opts; | ||
214 | + if (typeof options != "object") { | ||
215 | + opts = $.extend({}, $.pnotify.defaults); | ||
216 | + opts.pnotify_text = options; | ||
217 | + } else { | ||
218 | + opts = $.extend({}, $.pnotify.defaults, options); | ||
219 | + if (opts['pnotify_animation'] instanceof Object) { | ||
220 | + opts['pnotify_animation'] = $.extend({ | ||
221 | + effect_in:$.pnotify.defaults.pnotify_animation, | ||
222 | + effect_out:$.pnotify.defaults.pnotify_animation | ||
223 | + }, opts['pnotify_animation']); | ||
224 | + } | ||
225 | + } | ||
226 | + | ||
227 | + if (opts.pnotify_before_init) { | ||
228 | + if (opts.pnotify_before_init(opts) === false) | ||
229 | + return null; | ||
230 | + } | ||
231 | + | ||
232 | + // This keeps track of the last element the mouse was over, so | ||
233 | + // mouseleave, mouseenter, etc can be called. | ||
234 | + var nonblock_last_elem; | ||
235 | + // This is used to pass events through the notice if it is non-blocking. | ||
236 | + var nonblock_pass = function(e, e_name) { | ||
237 | + pnotify.css("display", "none"); | ||
238 | + var element_below = document.elementFromPoint(e.clientX, e.clientY); | ||
239 | + pnotify.css("display", "block"); | ||
240 | + var jelement_below = $(element_below); | ||
241 | + var cursor_style = jelement_below.css("cursor"); | ||
242 | + pnotify.css("cursor", cursor_style != "auto" ? cursor_style : "default"); | ||
243 | + // If the element changed, call mouseenter, mouseleave, etc. | ||
244 | + if (!nonblock_last_elem || nonblock_last_elem.get(0) != element_below) { | ||
245 | + if (nonblock_last_elem) { | ||
246 | + dom_event.call(nonblock_last_elem.get(0), "mouseleave", e.originalEvent); | ||
247 | + dom_event.call(nonblock_last_elem.get(0), "mouseout", e.originalEvent); | ||
248 | + } | ||
249 | + dom_event.call(element_below, "mouseenter", e.originalEvent); | ||
250 | + dom_event.call(element_below, "mouseover", e.originalEvent); | ||
251 | + } | ||
252 | + dom_event.call(element_below, e_name, e.originalEvent); | ||
253 | + // Remember the latest element the mouse was over. | ||
254 | + nonblock_last_elem = jelement_below; | ||
255 | + }; | ||
256 | + | ||
257 | + // Create our widget. | ||
258 | + // Stop animation, reset the removal timer, and show the close | ||
259 | + // button when the user mouses over. | ||
260 | + var pnotify = $14("<div />", { | ||
261 | + "class": "rf-ny " + opts.pnotify_addclass, | ||
262 | + "css": {"display": "none"}, | ||
263 | + "mouseenter": function(e) { | ||
264 | + if (opts.pnotify_nonblock) e.stopPropagation(); | ||
265 | + if (opts.pnotify_mouse_reset && animating == "out") { | ||
266 | + // If it's animating out, animate back in really quick. | ||
267 | + pnotify.stop(true); | ||
268 | + pnotify.css("height", "auto").animate({"width": opts.pnotify_width, "opacity": opts.pnotify_nonblock ? opts.pnotify_nonblock_opacity : opts.pnotify_opacity}, "fast"); | ||
269 | + } else if (opts.pnotify_nonblock && animating != "out") { | ||
270 | + // If it's non-blocking, animate to the other opacity. | ||
271 | + pnotify.animate({"opacity": opts.pnotify_nonblock_opacity}, "fast"); | ||
272 | + } | ||
273 | + if (opts.pnotify_hide && opts.pnotify_mouse_reset) pnotify.pnotify_cancel_remove(); | ||
274 | + if (opts.pnotify_closer && !opts.pnotify_nonblock) pnotify.closer.show(); | ||
275 | + }, | ||
276 | + "mouseleave": function(e) { | ||
277 | + if (opts.pnotify_nonblock) e.stopPropagation(); | ||
278 | + nonblock_last_elem = null; | ||
279 | + pnotify.css("cursor", "auto"); | ||
280 | + if (opts.pnotify_nonblock && animating != "out") | ||
281 | + pnotify.animate({"opacity": opts.pnotify_opacity}, "fast"); | ||
282 | + if (opts.pnotify_hide && opts.pnotify_mouse_reset) pnotify.pnotify_queue_remove(); | ||
283 | + pnotify.closer.hide(); | ||
284 | + $.pnotify_position_all(); | ||
285 | + }, | ||
286 | + "mouseover": function(e) { | ||
287 | + if (opts.pnotify_nonblock) e.stopPropagation(); | ||
288 | + }, | ||
289 | + "mouseout": function(e) { | ||
290 | + if (opts.pnotify_nonblock) e.stopPropagation(); | ||
291 | + }, | ||
292 | + "mousemove": function(e) { | ||
293 | + if (opts.pnotify_nonblock) { | ||
294 | + e.stopPropagation(); | ||
295 | + nonblock_pass(e, "onmousemove"); | ||
296 | + } | ||
297 | + }, | ||
298 | + "mousedown": function(e) { | ||
299 | + if (opts.pnotify_nonblock) { | ||
300 | + e.stopPropagation(); | ||
301 | + e.preventDefault(); | ||
302 | + nonblock_pass(e, "onmousedown"); | ||
303 | + } | ||
304 | + }, | ||
305 | + "mouseup": function(e) { | ||
306 | + if (opts.pnotify_nonblock) { | ||
307 | + e.stopPropagation(); | ||
308 | + e.preventDefault(); | ||
309 | + nonblock_pass(e, "onmouseup"); | ||
310 | + } | ||
311 | + }, | ||
312 | + "click": function(e) { | ||
313 | + if (opts.pnotify_nonblock) { | ||
314 | + e.stopPropagation(); | ||
315 | + nonblock_pass(e, "onclick"); | ||
316 | + } | ||
317 | + }, | ||
318 | + "dblclick": function(e) { | ||
319 | + if (opts.pnotify_nonblock) { | ||
320 | + e.stopPropagation(); | ||
321 | + nonblock_pass(e, "ondblclick"); | ||
322 | + } | ||
323 | + } | ||
324 | + }); | ||
325 | + pnotify.opts = opts; | ||
326 | + // Create a drop shadow. | ||
327 | + if (opts.pnotify_shadow && !$.browser.msie) | ||
328 | + pnotify.shadow_container = $14("<div />", {"class": "rf-ny-sh"}).prependTo(pnotify); | ||
329 | + // Create a container for the notice contents. | ||
330 | + pnotify.container = $14("<div />", {"class": "rf-ny-co"}) | ||
331 | + .appendTo(pnotify); | ||
332 | + | ||
333 | + pnotify.pnotify_version = "1.0.0"; | ||
334 | + | ||
335 | + // This function is for updating the notice. | ||
336 | + pnotify.pnotify = function(options) { | ||
337 | + // Update the notice. | ||
338 | + var old_opts = opts; | ||
339 | + if (typeof options == "string") | ||
340 | + opts.pnotify_text = options; | ||
341 | + else | ||
342 | + opts = $.extend({}, opts, options); | ||
343 | + pnotify.opts = opts; | ||
344 | + // Update the shadow. | ||
345 | + if (opts.pnotify_shadow != old_opts.pnotify_shadow) { | ||
346 | + if (opts.pnotify_shadow && !$.browser.msie) | ||
347 | + pnotify.shadow_container = $14("<div />", {"class": "rf-ny-sh"}).prependTo(pnotify); | ||
348 | + else | ||
349 | + pnotify.children(".rf-ny-sh").remove(); | ||
350 | + } | ||
351 | + // Update the additional classes. | ||
352 | + if (opts.pnotify_addclass === false) | ||
353 | + pnotify.removeClass(old_opts.pnotify_addclass); | ||
354 | + else if (opts.pnotify_addclass !== old_opts.pnotify_addclass) | ||
355 | + pnotify.removeClass(old_opts.pnotify_addclass).addClass(opts.pnotify_addclass); | ||
356 | + // Update the title. | ||
357 | + if (opts.pnotify_title === false) | ||
358 | + pnotify.title_container.hide("fast"); | ||
359 | + else if (opts.pnotify_title !== old_opts.pnotify_title) | ||
360 | + pnotify.title_container.html(opts.pnotify_title).show(200); | ||
361 | + // Update the text. | ||
362 | + if (opts.pnotify_text === false) { | ||
363 | + pnotify.text_container.hide("fast"); | ||
364 | + } else if (opts.pnotify_text !== old_opts.pnotify_text) { | ||
365 | + if (opts.pnotify_insert_brs) | ||
366 | + opts.pnotify_text = opts.pnotify_text.replace(/\n/g, "<br />"); | ||
367 | + pnotify.text_container.html(opts.pnotify_text).show(200); | ||
368 | + } | ||
369 | + pnotify.pnotify_history = opts.pnotify_history; | ||
370 | + // Change the notice type. | ||
371 | + if (opts.pnotify_type != old_opts.pnotify_type) | ||
372 | + pnotify.container.toggleClass("rf-ny-co rf-ny-co-hover"); | ||
373 | + if ((opts.pnotify_notice_icon != old_opts.pnotify_notice_icon && opts.pnotify_type == "notice") || | ||
374 | + (opts.pnotify_error_icon != old_opts.pnotify_error_icon && opts.pnotify_type == "error") || | ||
375 | + (opts.pnotify_type != old_opts.pnotify_type)) { | ||
376 | + // Remove any old icon. | ||
377 | + pnotify.container.find("div.rf-ny-ic").remove(); | ||
378 | + // if ((opts.pnotify_error_icon && opts.pnotify_type == "error") || (opts.pnotify_notice_icon)) { | ||
379 | + // Build the new icon. | ||
380 | + $14("<div />", {"class": "rf-ny-ic"}) | ||
381 | + .append($14("<span />", {"class": opts.pnotify_type == "error" ? opts.pnotify_error_icon : opts.pnotify_notice_icon})) | ||
382 | + .prependTo(pnotify.container); | ||
383 | + // } | ||
384 | + } | ||
385 | + // Update the width. | ||
386 | + if (opts.pnotify_width !== old_opts.pnotify_width) | ||
387 | + pnotify.animate({width: opts.pnotify_width}); | ||
388 | + // Update the minimum height. | ||
389 | + if (opts.pnotify_min_height !== old_opts.pnotify_min_height) | ||
390 | + pnotify.container.animate({minHeight: opts.pnotify_min_height}); | ||
391 | + // Update the opacity. | ||
392 | + if (opts.pnotify_opacity !== old_opts.pnotify_opacity) | ||
393 | + pnotify.fadeTo(opts.pnotify_animate_speed, opts.pnotify_opacity); | ||
394 | + if (!opts.pnotify_hide) | ||
395 | + pnotify.pnotify_cancel_remove(); | ||
396 | + else if (!old_opts.pnotify_hide) | ||
397 | + pnotify.pnotify_queue_remove(); | ||
398 | + pnotify.pnotify_queue_position(); | ||
399 | + return pnotify; | ||
400 | + }; | ||
401 | + | ||
402 | + // Queue the position function so it doesn't run repeatedly and use | ||
403 | + // up resources. | ||
404 | + pnotify.pnotify_queue_position = function() { | ||
405 | + if (timer) | ||
406 | + clearTimeout(timer); | ||
407 | + timer = setTimeout($.pnotify_position_all, 10); | ||
408 | + }; | ||
409 | + | ||
410 | + // Display the notice. | ||
411 | + pnotify.pnotify_display = function() { | ||
412 | + // If the notice is not in the DOM, append it. | ||
413 | + if (!pnotify.parent().length) | ||
414 | + pnotify.appendTo(body); | ||
415 | + // Run callback. | ||
416 | + if (opts.pnotify_before_open) { | ||
417 | + if (opts.pnotify_before_open(pnotify) === false) | ||
418 | + return; | ||
419 | + } | ||
420 | + pnotify.pnotify_queue_position(); | ||
421 | + // First show it, then set its opacity, then hide it. | ||
422 | + if (opts.pnotify_animation == "fade" || opts.pnotify_animation.effect_in == "fade") { | ||
423 | + // If it's fading in, it should start at 0. | ||
424 | + pnotify.show().fadeTo(0, 0).hide(); | ||
425 | + } else { | ||
426 | + // Or else it should be set to the opacity. | ||
427 | + if (opts.pnotify_opacity != 1) | ||
428 | + pnotify.show().fadeTo(0, opts.pnotify_opacity).hide(); | ||
429 | + } | ||
430 | + pnotify.animate_in(function() { | ||
431 | + if (opts.pnotify_after_open) | ||
432 | + opts.pnotify_after_open(pnotify); | ||
433 | + | ||
434 | + pnotify.pnotify_queue_position(); | ||
435 | + | ||
436 | + // Now set it to hide. | ||
437 | + if (opts.pnotify_hide) | ||
438 | + pnotify.pnotify_queue_remove(); | ||
439 | + }); | ||
440 | + }; | ||
441 | + | ||
442 | + // Remove the notice. | ||
443 | + pnotify.pnotify_remove = function() { | ||
444 | + if (pnotify.timer) { | ||
445 | + window.clearTimeout(pnotify.timer); | ||
446 | + pnotify.timer = null; | ||
447 | + } | ||
448 | + // Run callback. | ||
449 | + if (opts.pnotify_before_close) { | ||
450 | + if (opts.pnotify_before_close(pnotify) === false) | ||
451 | + return; | ||
452 | + } | ||
453 | + pnotify.animate_out(function() { | ||
454 | + if (opts.pnotify_after_close) { | ||
455 | + if (opts.pnotify_after_close(pnotify) === false) | ||
456 | + return; | ||
457 | + } | ||
458 | + pnotify.pnotify_queue_position(); | ||
459 | + // If we're supposed to remove the notice from the DOM, do it. | ||
460 | + if (opts.pnotify_remove) { | ||
461 | + if (opts.pnotify_history) { | ||
462 | + if (pnotify[0].parentNode != null) { | ||
463 | + pnotify[0].parentNode.removeChild(pnotify[0]); | ||
464 | + } | ||
465 | + } else { | ||
466 | + pnotify.remove(); | ||
467 | + } | ||
468 | + } | ||
469 | + }); | ||
470 | + }; | ||
471 | + | ||
472 | + // Animate the notice in. | ||
473 | + pnotify.animate_in = function(callback) { | ||
474 | + // Declare that the notice is animating in. (Or has completed animating in.) | ||
475 | + animating = "in"; | ||
476 | + var animation; | ||
477 | + if (typeof opts.pnotify_animation.effect_in != "undefined") | ||
478 | + animation = opts.pnotify_animation.effect_in; | ||
479 | + else | ||
480 | + animation = opts.pnotify_animation; | ||
481 | + if (animation == "none") { | ||
482 | + pnotify.show(); | ||
483 | + callback(); | ||
484 | + } else if (animation == "show") | ||
485 | + pnotify.show(opts.pnotify_animate_speed, callback); | ||
486 | + else if (animation == "fade") | ||
487 | + pnotify.show().fadeTo(opts.pnotify_animate_speed, opts.pnotify_opacity, callback); | ||
488 | + else if (animation == "slide") | ||
489 | + pnotify.slideDown(opts.pnotify_animate_speed, callback); | ||
490 | + else if (typeof animation == "function") | ||
491 | + animation("in", callback, pnotify); | ||
492 | + else if (pnotify.effect) | ||
493 | + pnotify.effect(animation, {}, opts.pnotify_animate_speed, callback); | ||
494 | + }; | ||
495 | + | ||
496 | + // Animate the notice out. | ||
497 | + pnotify.animate_out = function(callback) { | ||
498 | + // Declare that the notice is animating out. (Or has completed animating out.) | ||
499 | + animating = "out"; | ||
500 | + var animation; | ||
501 | + if (typeof opts.pnotify_animation.effect_out != "undefined") | ||
502 | + animation = opts.pnotify_animation.effect_out; | ||
503 | + else | ||
504 | + animation = opts.pnotify_animation; | ||
505 | + if (animation == "none") { | ||
506 | + pnotify.hide(); | ||
507 | + callback(); | ||
508 | + } else if (animation == "show") | ||
509 | + pnotify.hide(opts.pnotify_animate_speed, callback); | ||
510 | + else if (animation == "fade") | ||
511 | + pnotify.fadeOut(opts.pnotify_animate_speed, callback); | ||
512 | + else if (animation == "slide") | ||
513 | + pnotify.slideUp(opts.pnotify_animate_speed, callback); | ||
514 | + else if (typeof animation == "function") | ||
515 | + animation("out", callback, pnotify); | ||
516 | + else if (pnotify.effect) | ||
517 | + pnotify.effect(animation, {}, opts.pnotify_animate_speed, callback); | ||
518 | + }; | ||
519 | + | ||
520 | + // Cancel any pending removal timer. | ||
521 | + pnotify.pnotify_cancel_remove = function() { | ||
522 | + if (pnotify.timer) | ||
523 | + window.clearTimeout(pnotify.timer); | ||
524 | + }; | ||
525 | + | ||
526 | + // Queue a removal timer. | ||
527 | + pnotify.pnotify_queue_remove = function() { | ||
528 | + // Cancel any current removal timer. | ||
529 | + pnotify.pnotify_cancel_remove(); | ||
530 | + pnotify.timer = window.setTimeout(function() { | ||
531 | + pnotify.pnotify_remove(); | ||
532 | + }, (isNaN(opts.pnotify_delay) ? 0 : opts.pnotify_delay)); | ||
533 | + }; | ||
534 | + | ||
535 | + // Provide a button to close the notice. | ||
536 | + pnotify.closer = $14("<div />", { | ||
537 | + "class": "rf-ny-cl", | ||
538 | + "css": {"cursor": "pointer", "display": "none"}, | ||
539 | + "click": function() { | ||
540 | + pnotify.pnotify_remove(); | ||
541 | + pnotify.closer.hide(); | ||
542 | + } | ||
543 | + }) | ||
544 | + .append($14("<span />", {"class": "rf-ny-cl-ic"})) | ||
545 | + .appendTo(pnotify.container); | ||
546 | + | ||
547 | + // Add the appropriate icon. | ||
548 | + // if ((opts.pnotify_error_icon && opts.pnotify_type == "error") || (opts.pnotify_notice_icon)) { | ||
549 | + $14("<div />", {"class": "rf-ny-ic"}) | ||
550 | + .append($14("<span />", {"class": opts.pnotify_type == "error" ? opts.pnotify_error_icon : opts.pnotify_notice_icon})) | ||
551 | + .appendTo(pnotify.container); | ||
552 | + // } | ||
553 | + | ||
554 | + // Add a title. | ||
555 | + pnotify.title_container = $14("<div />", { | ||
556 | + "class": "rf-ny-tl", | ||
557 | + "html": opts.pnotify_title | ||
558 | + }) | ||
559 | + .appendTo(pnotify.container); | ||
560 | + if (opts.pnotify_title === false) | ||
561 | + pnotify.title_container.hide(); | ||
562 | + | ||
563 | + // Replace new lines with HTML line breaks. | ||
564 | + if (opts.pnotify_insert_brs && typeof opts.pnotify_text == "string") | ||
565 | + opts.pnotify_text = opts.pnotify_text.replace(/\n/g, "<br />"); | ||
566 | + // Add text. | ||
567 | + pnotify.text_container = $14("<div />", { | ||
568 | + "class": "rf-ny-te", | ||
569 | + "html": opts.pnotify_text | ||
570 | + }) | ||
571 | + .appendTo(pnotify.container); | ||
572 | + if (opts.pnotify_text === false) | ||
573 | + pnotify.text_container.hide(); | ||
574 | + | ||
575 | + // Set width and min height. | ||
576 | + if (typeof opts.pnotify_width == "string") | ||
577 | + pnotify.css("width", opts.pnotify_width); | ||
578 | + if (typeof opts.pnotify_min_height == "string") | ||
579 | + pnotify.container.css("min-height", opts.pnotify_min_height); | ||
580 | + | ||
581 | + // The history variable controls whether the notice gets redisplayed | ||
582 | + // by the history pull down. | ||
583 | + pnotify.pnotify_history = opts.pnotify_history; | ||
584 | + | ||
585 | + // Add the notice to the notice array. | ||
586 | + var body_data = body.data("pnotify"); | ||
587 | + if (body_data == null || typeof body_data != "object") | ||
588 | + body_data = []; | ||
589 | + if (opts.pnotify_stack.push == "top") | ||
590 | + body_data = $.merge([pnotify], body_data); | ||
591 | + else | ||
592 | + body_data = $.merge(body_data, [pnotify]); | ||
593 | + body.data("pnotify", body_data); | ||
594 | + | ||
595 | + // Run callback. | ||
596 | + if (opts.pnotify_after_init) | ||
597 | + opts.pnotify_after_init(pnotify); | ||
598 | + | ||
599 | + if (opts.pnotify_history) { | ||
600 | + // If there isn't a history pull down, create one. | ||
601 | + var body_history = body.data("pnotify_history"); | ||
602 | + if (typeof body_history == "undefined") { | ||
603 | + body_history = $14("<div />", { | ||
604 | + "class": "rf-ny-hc", | ||
605 | + "mouseleave": function() { | ||
606 | + body_history.animate({top: "-" + history_handle_top + "px"}, {duration: 100, queue: false}); | ||
607 | + } | ||
608 | + }) | ||
609 | + .append($14("<div />", {"class": "rf-ny-hh", "text": "Redisplay"})) | ||
610 | + .append($14("<button />", { | ||
611 | + "class": "rf-ny-ha", | ||
612 | + "text": "All", | ||
613 | + // "mouseenter": function(){ | ||
614 | + // $(this).addClass("ui-state-hover"); | ||
615 | + // }, | ||
616 | + // "mouseleave": function(){ | ||
617 | + // $(this).removeClass("ui-state-hover"); | ||
618 | + // }, | ||
619 | + "click": function() { | ||
620 | + // Display all notices. (Disregarding non-history notices.) | ||
621 | + $.each(body.data("pnotify"), function() { | ||
622 | + if (this.pnotify_history && this.pnotify_display) | ||
623 | + this.pnotify_display(); | ||
624 | + }); | ||
625 | + return false; | ||
626 | + } | ||
627 | + })) | ||
628 | + .append($14("<button />", { | ||
629 | + "class": "rf-ny-hl", | ||
630 | + "text": "Last", | ||
631 | + // "mouseenter": function(){ | ||
632 | + // $(this).addClass("ui-state-hover"); | ||
633 | + // }, | ||
634 | + // "mouseleave": function(){ | ||
635 | + // $(this).removeClass("ui-state-hover"); | ||
636 | + // }, | ||
637 | + "click": function() { | ||
638 | + // Look up the last history notice, and display it. | ||
639 | + var i = 1; | ||
640 | + var body_data = body.data("pnotify"); | ||
641 | + while (!body_data[body_data.length - i] || !body_data[body_data.length - i].pnotify_history || body_data[body_data.length - i].is(":visible")) { | ||
642 | + if (body_data.length - i === 0) | ||
643 | + return false; | ||
644 | + i++; | ||
645 | + } | ||
646 | + var n = body_data[body_data.length - i]; | ||
647 | + if (n.pnotify_display) | ||
648 | + n.pnotify_display(); | ||
649 | + return false; | ||
650 | + } | ||
651 | + })) | ||
652 | + .appendTo(body); | ||
653 | + | ||
654 | + // Make a handle so the user can pull down the history pull down. | ||
655 | + var handle = $14("<span />", { | ||
656 | + "class": "rf-ny-hp", | ||
657 | + "mouseenter": function() { | ||
658 | + body_history.animate({top: "0"}, {duration: 100, queue: false}); | ||
659 | + } | ||
660 | + }) | ||
661 | + .appendTo(body_history); | ||
662 | + | ||
663 | + // Get the top of the handle. | ||
664 | + history_handle_top = handle.offset().top + 2; | ||
665 | + // Hide the history pull down up to the top of the handle. | ||
666 | + body_history.css({top: "-" + history_handle_top + "px"}); | ||
667 | + // Save the history pull down. | ||
668 | + body.data("pnotify_history", body_history); | ||
669 | + } | ||
670 | + } | ||
671 | + | ||
672 | + // Mark the stack so it won't animate the new notice. | ||
673 | + opts.pnotify_stack.animation = false; | ||
674 | + | ||
675 | + // Display the notice. | ||
676 | + pnotify.pnotify_display(); | ||
677 | + | ||
678 | + return pnotify; | ||
679 | + } | ||
680 | + }); | ||
681 | + | ||
682 | + // Some useful regexes. | ||
683 | + var re_on = /^on/; | ||
684 | + var re_mouse_events = /^(dbl)?click$|^mouse(move|down|up|over|out|enter|leave)$|^contextmenu$/; | ||
685 | + var re_ui_events = /^(focus|blur|select|change|reset)$|^key(press|down|up)$/; | ||
686 | + var re_html_events = /^(scroll|resize|(un)?load|abort|error)$/; | ||
687 | + // Fire a DOM event. | ||
688 | + var dom_event = function(e, orig_e) { | ||
689 | + var event_object; | ||
690 | + e = e.toLowerCase(); | ||
691 | + if (document.createEvent && this.dispatchEvent) { | ||
692 | + // FireFox, Opera, Safari, Chrome | ||
693 | + e = e.replace(re_on, ''); | ||
694 | + if (e.match(re_mouse_events)) { | ||
695 | + // This allows the click event to fire on the notice. There is | ||
696 | + // probably a much better way to do it. | ||
697 | + $(this).offset(); | ||
698 | + event_object = document.createEvent("MouseEvents"); | ||
699 | + event_object.initMouseEvent( | ||
700 | + e, orig_e.bubbles, orig_e.cancelable, orig_e.view, orig_e.detail, | ||
701 | + orig_e.screenX, orig_e.screenY, orig_e.clientX, orig_e.clientY, | ||
702 | + orig_e.ctrlKey, orig_e.altKey, orig_e.shiftKey, orig_e.metaKey, orig_e.button, orig_e.relatedTarget | ||
703 | + ); | ||
704 | + } else if (e.match(re_ui_events)) { | ||
705 | + event_object = document.createEvent("UIEvents"); | ||
706 | + event_object.initUIEvent(e, orig_e.bubbles, orig_e.cancelable, orig_e.view, orig_e.detail); | ||
707 | + } else if (e.match(re_html_events)) { | ||
708 | + event_object = document.createEvent("HTMLEvents"); | ||
709 | + event_object.initEvent(e, orig_e.bubbles, orig_e.cancelable); | ||
710 | + } | ||
711 | + if (!event_object) return; | ||
712 | + this.dispatchEvent(event_object); | ||
713 | + } else { | ||
714 | + // Internet Explorer | ||
715 | + if (!e.match(re_on)) e = "on" + e; | ||
716 | + event_object = document.createEventObject(orig_e); | ||
717 | + this.fireEvent(e, event_object); | ||
718 | + } | ||
719 | + }; | ||
720 | + | ||
721 | + $.pnotify.defaults = { | ||
722 | + // The notice's title. | ||
723 | + pnotify_title: false, | ||
724 | + // The notice's text. | ||
725 | + pnotify_text: false, | ||
726 | + // Additional classes to be added to the notice. (For custom styling.) | ||
727 | + pnotify_addclass: "", | ||
728 | + // Create a non-blocking notice. It lets the user click elements underneath it. | ||
729 | + pnotify_nonblock: false, | ||
730 | + // The opacity of the notice (if it's non-blocking) when the mouse is over it. | ||
731 | + pnotify_nonblock_opacity: .2, | ||
732 | + // Display a pull down menu to redisplay previous notices, and place the notice in the history. | ||
733 | + pnotify_history: true, | ||
734 | + // Width of the notice. | ||
735 | + pnotify_width: "300px", | ||
736 | + // Minimum height of the notice. It will expand to fit content. | ||
737 | + pnotify_min_height: "16px", | ||
738 | + // Type of the notice. "notice" or "error". | ||
739 | + pnotify_type: "notice", | ||
740 | + // The icon class to use if type is notice. | ||
741 | + pnotify_notice_icon: "", | ||
742 | + // The icon class to use if type is error. | ||
743 | + pnotify_error_icon: "", | ||
744 | + // The animation to use when displaying and hiding the notice. "none", "show", "fade", and "slide" are built in to jQuery. Others require jQuery UI. Use an object with effect_in and effect_out to use different effects. | ||
745 | + pnotify_animation: "fade", | ||
746 | + // Speed at which the notice animates in and out. "slow", "def" or "normal", "fast" or number of milliseconds. | ||
747 | + pnotify_animate_speed: "slow", | ||
748 | + // Opacity of the notice. | ||
749 | + pnotify_opacity: 1, | ||
750 | + // Display a drop shadow. | ||
751 | + pnotify_shadow: false, | ||
752 | + // Provide a button for the user to manually close the notice. | ||
753 | + pnotify_closer: true, | ||
754 | + // After a delay, remove the notice. | ||
755 | + pnotify_hide: true, | ||
756 | + // Delay in milliseconds before the notice is removed. | ||
757 | + pnotify_delay: 8000, | ||
758 | + // Reset the hide timer if the mouse moves over the notice. | ||
759 | + pnotify_mouse_reset: true, | ||
760 | + // Remove the notice's elements from the DOM after it is removed. | ||
761 | + pnotify_remove: true, | ||
762 | + // Change new lines to br tags. | ||
763 | + pnotify_insert_brs: true, | ||
764 | + // The stack on which the notices will be placed. Also controls the direction the notices stack. | ||
765 | + pnotify_stack: {"dir1": "down", "dir2": "left", "push": "bottom"} | ||
766 | + }; | ||
767 | +})(jQuery); |
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" | ||
2 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
3 | + xmlns:f="http://java.sun.com/jsf/core" | ||
4 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
5 | + <div> | ||
6 | + <ul> | ||
7 | + <!--<li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/sample_1.jsf">Example 1</h:outputLink></li>--> | ||
8 | + </ul> | ||
9 | + </div> | ||
10 | +</ui:component> |
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" | ||
4 | + xmlns:f="http://java.sun.com/jsf/core" | ||
5 | + xmlns:h="http://java.sun.com/jsf/html" xmlns:sandbox="http://richfaces.org/sandbox/jQueryPlugin" | ||
6 | + xmlns:a4j="http://richfaces.org/a4j" | ||
7 | + xmlns:rich="http://richfaces.org/rich"> | ||
8 | +<head> | ||
9 | + <title>jQueryPlugin sample</title> | ||
10 | + <a4j:loadStyle src="resource:///jquery.pnotify.xcss"/> | ||
11 | + <style type="text/css"> | ||
12 | + #calendar { | ||
13 | + float: right; | ||
14 | + width: 200px; | ||
15 | + } | ||
16 | + </style> | ||
17 | +</head> | ||
18 | + | ||
19 | +<body> | ||
20 | +<ui:include src="menu.xhtml"/> | ||
21 | + | ||
22 | +<p> | ||
23 | + This sample shows how to load 2 different jQuery plugins </p> | ||
24 | +<ul> | ||
25 | + <li>pnotify</li> | ||
26 | + <li>fullCalendar</li> | ||
27 | +</ul> | ||
28 | + | ||
29 | +<div id="calendar"></div> | ||
30 | + | ||
31 | +<script type="text/javascript"> | ||
32 | + jQuery("#calendar").fullCalendar({defaultView: 'month'}); | ||
33 | + jQuery.pnotify({pnotify_title:'Hello!',pnotify_text:'I\'m a custom plugin',pnotify_hide:false}); | ||
34 | +</script> | ||
35 | + | ||
36 | +<sandbox:jQueryPlugin src="/jquery.pnotify.js"/> | ||
37 | +<sandbox:jQueryPlugin src="/fullcalendar.js"/> | ||
38 | + | ||
39 | +<rich:insert src="/sample_1.xhtml" highlight="html"/> | ||
40 | + | ||
41 | +</body> | ||
42 | +</html> |
samples/lightbox-sample/pom.xml
0 → 100644
1 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
2 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
3 | + <parent> | ||
4 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
5 | + <artifactId>parent</artifactId> | ||
6 | + <version>3.3.4-SNAPSHOT</version> | ||
7 | + <relativePath>../pom.xml</relativePath> | ||
8 | + </parent> | ||
9 | + <modelVersion>4.0.0</modelVersion> | ||
10 | + <groupId>org.richfaces</groupId> | ||
11 | + <artifactId>lightbox-sample</artifactId> | ||
12 | + <packaging>war</packaging> | ||
13 | + <name>lightbox-sample Maven Webapp</name> | ||
14 | + <build> | ||
15 | + <finalName>lightbox-sample</finalName> | ||
16 | + </build> | ||
17 | + <dependencies> | ||
18 | + <dependency> | ||
19 | + <groupId>org.richfaces.ui</groupId> | ||
20 | + <artifactId>core</artifactId> | ||
21 | + <version>${project.version}</version> | ||
22 | + </dependency> | ||
23 | + <dependency> | ||
24 | + <groupId>org.richfaces.ui</groupId> | ||
25 | + <artifactId>richfaces-ui</artifactId> | ||
26 | + </dependency> | ||
27 | + <dependency> | ||
28 | + <groupId>com.uwyn</groupId> | ||
29 | + <artifactId>jhighlight</artifactId> | ||
30 | + <version>1.0</version> | ||
31 | + </dependency> | ||
32 | + <dependency> | ||
33 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
34 | + <artifactId>lightbox</artifactId> | ||
35 | + <version>${project.version}</version> | ||
36 | + </dependency> | ||
37 | + </dependencies> | ||
38 | +</project> |
1 | +package org.richfaces.samples.lightbox; | ||
2 | + | ||
3 | +import java.io.Serializable; | ||
4 | + | ||
5 | +public class ConfigBean implements Serializable { | ||
6 | + | ||
7 | + private String overlayBgColor = "#000"; | ||
8 | + private double overlayOpacity = .8; | ||
9 | + private int containerBorderSize = 10; | ||
10 | + private int containerResizeSpeed = 400; | ||
11 | + private boolean fixedNavigation = false; | ||
12 | + private String keyToClose = "c"; | ||
13 | + private String keyToNext = "n"; | ||
14 | + private String keyToPrev = "p"; | ||
15 | + private String txtImage = "Image"; | ||
16 | + private String txtOf = "of"; | ||
17 | + private String imageBlank; | ||
18 | + private String imageBtnClose; | ||
19 | + private String imageBtnNext; | ||
20 | + private String imageBtnPrev; | ||
21 | + private String imageLoading; | ||
22 | + | ||
23 | + public String getOverlayBgColor() { | ||
24 | + return overlayBgColor; | ||
25 | + } | ||
26 | + | ||
27 | + public void setOverlayBgColor(String overlayBgColor) { | ||
28 | + this.overlayBgColor = overlayBgColor; | ||
29 | + } | ||
30 | + | ||
31 | + public double getOverlayOpacity() { | ||
32 | + return overlayOpacity; | ||
33 | + } | ||
34 | + | ||
35 | + public void setOverlayOpacity(double overlayOpacity) { | ||
36 | + this.overlayOpacity = overlayOpacity; | ||
37 | + } | ||
38 | + | ||
39 | + public int getContainerBorderSize() { | ||
40 | + return containerBorderSize; | ||
41 | + } | ||
42 | + | ||
43 | + public void setContainerBorderSize(int containerBorderSize) { | ||
44 | + this.containerBorderSize = containerBorderSize; | ||
45 | + } | ||
46 | + | ||
47 | + public int getContainerResizeSpeed() { | ||
48 | + return containerResizeSpeed; | ||
49 | + } | ||
50 | + | ||
51 | + public void setContainerResizeSpeed(int containerResizeSpeed) { | ||
52 | + this.containerResizeSpeed = containerResizeSpeed; | ||
53 | + } | ||
54 | + | ||
55 | + public boolean isFixedNavigation() { | ||
56 | + return fixedNavigation; | ||
57 | + } | ||
58 | + | ||
59 | + public void setFixedNavigation(boolean fixedNavigation) { | ||
60 | + this.fixedNavigation = fixedNavigation; | ||
61 | + } | ||
62 | + | ||
63 | + public String getKeyToClose() { | ||
64 | + return keyToClose; | ||
65 | + } | ||
66 | + | ||
67 | + public void setKeyToClose(String keyToClose) { | ||
68 | + this.keyToClose = keyToClose; | ||
69 | + } | ||
70 | + | ||
71 | + public String getKeyToNext() { | ||
72 | + return keyToNext; | ||
73 | + } | ||
74 | + | ||
75 | + public void setKeyToNext(String keyToNext) { | ||
76 | + this.keyToNext = keyToNext; | ||
77 | + } | ||
78 | + | ||
79 | + public String getKeyToPrev() { | ||
80 | + return keyToPrev; | ||
81 | + } | ||
82 | + | ||
83 | + public void setKeyToPrev(String keyToPrev) { | ||
84 | + this.keyToPrev = keyToPrev; | ||
85 | + } | ||
86 | + | ||
87 | + public String getTxtImage() { | ||
88 | + return txtImage; | ||
89 | + } | ||
90 | + | ||
91 | + public void setTxtImage(String txtImage) { | ||
92 | + this.txtImage = txtImage; | ||
93 | + } | ||
94 | + | ||
95 | + public String getTxtOf() { | ||
96 | + return txtOf; | ||
97 | + } | ||
98 | + | ||
99 | + public void setTxtOf(String txtOf) { | ||
100 | + this.txtOf = txtOf; | ||
101 | + } | ||
102 | + | ||
103 | + public void setImageBlank(String imageBlank) { | ||
104 | + this.imageBlank = imageBlank; | ||
105 | + } | ||
106 | + | ||
107 | + public String getImageBlank() { | ||
108 | + return imageBlank; | ||
109 | + } | ||
110 | + | ||
111 | + public void setImageBtnClose(String imageBtnClose) { | ||
112 | + this.imageBtnClose = imageBtnClose; | ||
113 | + } | ||
114 | + | ||
115 | + public String getImageBtnClose() { | ||
116 | + return imageBtnClose; | ||
117 | + } | ||
118 | + | ||
119 | + public void setImageBtnNext(String imageBtnNext) { | ||
120 | + this.imageBtnNext = imageBtnNext; | ||
121 | + } | ||
122 | + | ||
123 | + public String getImageBtnNext() { | ||
124 | + return imageBtnNext; | ||
125 | + } | ||
126 | + | ||
127 | + public void setImageBtnPrev(String imageBtnPrev) { | ||
128 | + this.imageBtnPrev = imageBtnPrev; | ||
129 | + } | ||
130 | + | ||
131 | + public String getImageBtnPrev() { | ||
132 | + return imageBtnPrev; | ||
133 | + } | ||
134 | + | ||
135 | + public void setImageLoading(String imageLoading) { | ||
136 | + this.imageLoading = imageLoading; | ||
137 | + } | ||
138 | + | ||
139 | + public String getImageLoading() { | ||
140 | + return imageLoading; | ||
141 | + } | ||
142 | +} |
1 | +<?xml version="1.0"?> | ||
2 | +<!DOCTYPE faces-config PUBLIC "-//Sun Microsystems, Inc.//DTD JavaServer Faces Config 1.0//EN" | ||
3 | + "http://java.sun.com/dtd/web-facesconfig_1_0.dtd"> | ||
4 | +<faces-config> | ||
5 | + <managed-bean> | ||
6 | + <managed-bean-name>config</managed-bean-name> | ||
7 | + <managed-bean-class>org.richfaces.samples.lightbox.ConfigBean</managed-bean-class> | ||
8 | + <managed-bean-scope>session</managed-bean-scope> | ||
9 | + </managed-bean> | ||
10 | +</faces-config> | ||
11 | + |
1 | +<jboss-deployment-structure> | ||
2 | + <deployment> | ||
3 | + <exclusions> | ||
4 | + <module name="javax.faces.api" slot="main"/> | ||
5 | + <module name="com.sun.jsf-impl" slot="main"/> | ||
6 | + </exclusions> | ||
7 | + <dependencies> | ||
8 | + <module name="org.apache.commons.logging"/> | ||
9 | + <module name="org.apache.commons.collections"/> | ||
10 | + <module name="org.apache.commons.beanutils"/> | ||
11 | + <module name="javax.faces.api" slot="1.2"/> | ||
12 | + <module name="com.sun.jsf-impl" slot="1.2"/> | ||
13 | + </dependencies> | ||
14 | + </deployment> | ||
15 | +</jboss-deployment-structure> | ||
16 | + |
1 | +<?xml version="1.0" encoding="UTF-8"?> | ||
2 | +<web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" | ||
3 | + xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
4 | + xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> | ||
5 | + <description>Richfaces lightbox Sample</description> | ||
6 | + <display-name>lightbox-sample</display-name> | ||
7 | + <context-param> | ||
8 | + <param-name>javax.faces.DEFAULT_SUFFIX</param-name> | ||
9 | + <param-value>.xhtml</param-value> | ||
10 | + </context-param> | ||
11 | + <context-param> | ||
12 | + <param-name>facelets.REFRESH_PERIOD</param-name> | ||
13 | + <param-value>2</param-value> | ||
14 | + </context-param> | ||
15 | + <context-param> | ||
16 | + <param-name>facelets.DEVELOPMENT</param-name> | ||
17 | + <param-value>true</param-value> | ||
18 | + </context-param> | ||
19 | + <context-param> | ||
20 | + <param-name>javax.faces.STATE_SAVING_METHOD</param-name> | ||
21 | + <param-value>client</param-value> | ||
22 | + </context-param> | ||
23 | + <context-param> | ||
24 | + <param-name>com.sun.faces.validateXml</param-name> | ||
25 | + <param-value>true</param-value> | ||
26 | + </context-param> | ||
27 | + <context-param> | ||
28 | + <param-name>com.sun.faces.verifyObjects</param-name> | ||
29 | + <param-value>true</param-value> | ||
30 | + </context-param> | ||
31 | + <context-param> | ||
32 | + <param-name>org.ajax4jsf.VIEW_HANDLERS</param-name> | ||
33 | + <param-value>com.sun.facelets.FaceletViewHandler</param-value> | ||
34 | + </context-param> | ||
35 | + <context-param> | ||
36 | + <param-name>org.ajax4jsf.COMPRESS_SCRIPT</param-name> | ||
37 | + <param-value>false</param-value> | ||
38 | + </context-param> | ||
39 | + <filter> | ||
40 | + <display-name>Ajax4jsf Filter</display-name> | ||
41 | + <filter-name>ajax4jsf</filter-name> | ||
42 | + <filter-class>org.ajax4jsf.Filter</filter-class> | ||
43 | + </filter> | ||
44 | + <filter-mapping> | ||
45 | + <filter-name>ajax4jsf</filter-name> | ||
46 | + <servlet-name>Faces Servlet</servlet-name> | ||
47 | + <dispatcher>FORWARD</dispatcher> | ||
48 | + <dispatcher>REQUEST</dispatcher> | ||
49 | + <dispatcher>INCLUDE</dispatcher> | ||
50 | + </filter-mapping> | ||
51 | + <servlet> | ||
52 | + <servlet-name>Faces Servlet</servlet-name> | ||
53 | + <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> | ||
54 | + <load-on-startup>1</load-on-startup> | ||
55 | + </servlet> | ||
56 | + <servlet-mapping> | ||
57 | + <servlet-name>Faces Servlet</servlet-name> | ||
58 | + <url-pattern>*.jsf</url-pattern> | ||
59 | + </servlet-mapping> | ||
60 | + <login-config> | ||
61 | + <auth-method>BASIC</auth-method> | ||
62 | + </login-config> | ||
63 | + <listener> | ||
64 | + | ||
65 | + <listener-class>com.sun.faces.config.ConfigureListener</listener-class> | ||
66 | + | ||
67 | + </listener> | ||
68 | +</web-app> |
1 | +<ui:component xmlns="http://www.w3.org/1999/xhtml" | ||
2 | + xmlns:ui="http://java.sun.com/jsf/facelets" | ||
3 | + xmlns:f="http://java.sun.com/jsf/core" | ||
4 | + xmlns:h="http://java.sun.com/jsf/html"> | ||
5 | + <div> | ||
6 | + <ul> | ||
7 | + <!--<li><h:outputLink value="#{facesContext.externalContext.requestContextPath}/sample_1.jsf">Example 1</h:outputLink></li>--> | ||
8 | + </ul> | ||
9 | + </div> | ||
10 | +</ui:component> |
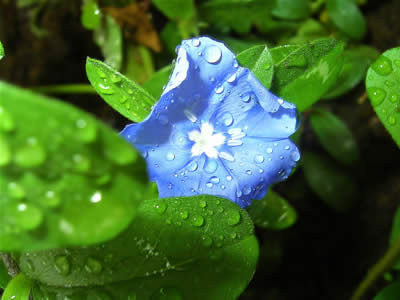
19.5 KB
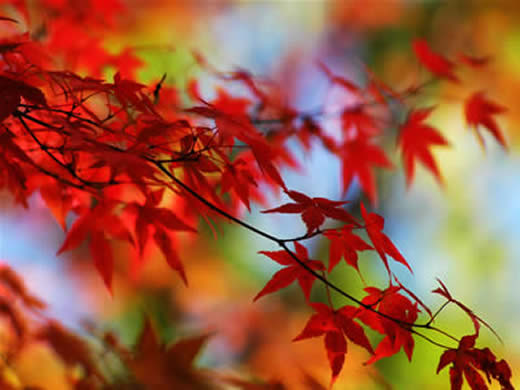
44.5 KB
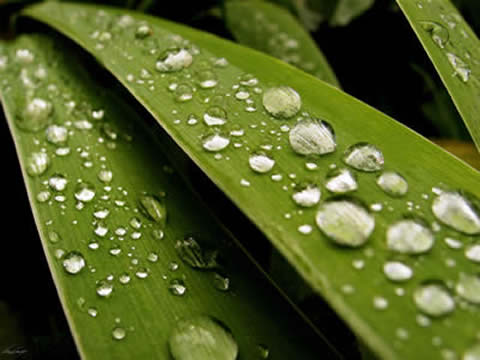
38.4 KB
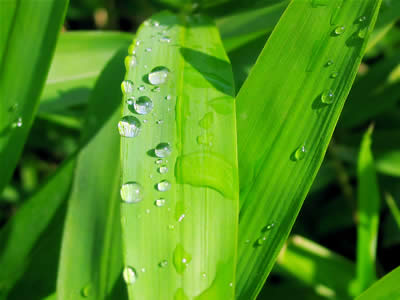
18.3 KB
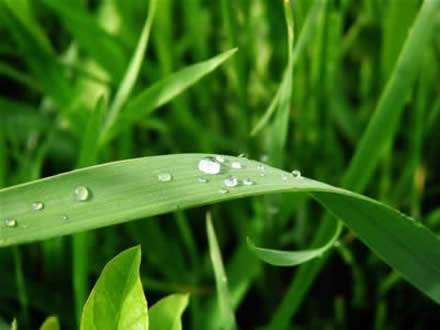
30.2 KB

2.04 KB

2.48 KB

2.18 KB

1.95 KB

2 KB
1 | +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" | ||
2 | + "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> | ||
3 | +<html xmlns="http://www.w3.org/1999/xhtml" xmlns:ui="http://java.sun.com/jsf/facelets" | ||
4 | + xmlns:f="http://java.sun.com/jsf/core" | ||
5 | + xmlns:h="http://java.sun.com/jsf/html" xmlns:lightbox="http://richfaces.org/sandbox/lightbox" | ||
6 | + xmlns:a4j="http://richfaces.org/a4j" | ||
7 | + xmlns:rich="http://richfaces.org/rich"> | ||
8 | +<head> | ||
9 | + <title>Lightbox sample</title> | ||
10 | + <style type="text/css"> | ||
11 | + #gallery ul { | ||
12 | + list-style: none outside none; | ||
13 | + } | ||
14 | + | ||
15 | + #gallery ul li { | ||
16 | + display: inline; | ||
17 | + } | ||
18 | + | ||
19 | + #gallery ul img { | ||
20 | + border: 5px solid #3E3E3E; | ||
21 | + border-bottom-width: 20px; | ||
22 | + } | ||
23 | + | ||
24 | + #gallery ul a:hover img { | ||
25 | + border: 5px solid #000; | ||
26 | + border-bottom-width: 20px; | ||
27 | + color: #FFFFFF; | ||
28 | + } | ||
29 | + | ||
30 | + #gallery ul a:hover { | ||
31 | + color: #FFFFFF; | ||
32 | + } | ||
33 | + | ||
34 | + </style> | ||
35 | +</head> | ||
36 | + | ||
37 | +<body> | ||
38 | +<ui:include src="menu.xhtml"/> | ||
39 | + | ||
40 | +<div id="gallery"> | ||
41 | + <ul> | ||
42 | + <li> | ||
43 | + <a href="photos/image1.jpg" title="Blue flower"> | ||
44 | + <h:graphicImage value="photos/thumb_image1.jpg"/> | ||
45 | + </a> | ||
46 | + </li> | ||
47 | + <li> | ||
48 | + <a href="photos/image2.jpg" title="Red leafs"> | ||
49 | + <h:graphicImage value="photos/thumb_image2.jpg"/> | ||
50 | + </a> | ||
51 | + </li> | ||
52 | + <li> | ||
53 | + <a href="photos/image3.jpg" title="Rain drops"> | ||
54 | + <h:graphicImage value="photos/thumb_image3.jpg"/> | ||
55 | + </a> | ||
56 | + </li> | ||
57 | + <li> | ||
58 | + <a href="photos/image4.jpg" title="Fresh green"> | ||
59 | + <h:graphicImage value="photos/thumb_image4.jpg"/> | ||
60 | + </a> | ||
61 | + </li> | ||
62 | + <li> | ||
63 | + <a href="photos/image5.jpg" title="More fresh green"> | ||
64 | + <h:graphicImage value="photos/thumb_image5.jpg"/> | ||
65 | + </a> | ||
66 | + </li> | ||
67 | + </ul> | ||
68 | +</div> | ||
69 | + | ||
70 | + | ||
71 | +<lightbox:lightbox id="lightbox" selector="#gallery a" containerBorderSize="#{config.containerBorderSize}" | ||
72 | + containerResizeSpeed="#{config.containerResizeSpeed}" keyToClose="#{config.keyToClose}" | ||
73 | + keyToNext="#{config.keyToNext}" keyToPrev="#{config.keyToPrev}" | ||
74 | + overlayBgColor="#{config.overlayBgColor}" | ||
75 | + overlayOpacity="#{config.overlayOpacity}" txtImage="#{config.txtImage}" txtOf="#{config.txtOf}" | ||
76 | + imageBlank="#{config.imageBlank}" | ||
77 | + imageBtnClose="#{config.imageBtnClose}" | ||
78 | + imageBtnNext="#{config.imageBtnNext}" | ||
79 | + imageBtnPrev="#{config.imageBtnPrev}" | ||
80 | + imageLoading="#{config.imageLoading}" | ||
81 | + /> | ||
82 | + | ||
83 | + | ||
84 | +<h:form id="lightboxForm"> | ||
85 | + | ||
86 | + | ||
87 | + <h:panelGrid columns="3"> | ||
88 | + <h:outputLabel value="containerBorderSize" for="containerBorderSize"/> | ||
89 | + <h:inputText id="containerBorderSize" value="#{config.containerBorderSize}"/> | ||
90 | + <h:message for="containerBorderSize"/> | ||
91 | + | ||
92 | + <h:outputLabel value="containerResizeSpeed" for="containerResizeSpeed"/> | ||
93 | + <h:inputText id="containerResizeSpeed" value="#{config.containerResizeSpeed}"/> | ||
94 | + <h:message for="containerResizeSpeed"/> | ||
95 | + | ||
96 | + <h:outputLabel value="keyToClose" for="keyToClose"/> | ||
97 | + <h:inputText id="keyToClose" value="#{config.keyToClose}"/> | ||
98 | + <h:message for="keyToClose"/> | ||
99 | + | ||
100 | + <h:outputLabel value="keyToNext" for="keyToNext"/> | ||
101 | + <h:inputText id="keyToNext" value="#{config.keyToNext}"/> | ||
102 | + <h:message for="keyToNext"/> | ||
103 | + | ||
104 | + <h:outputLabel value="" for="keyToPrev"/> | ||
105 | + <h:inputText id="keyToPrev" value="#{config.keyToPrev}"/> | ||
106 | + <h:message for="keyToPrev"/> | ||
107 | + | ||
108 | + <h:outputLabel value="overlayBgColor" for="overlayBgColor"/> | ||
109 | + <h:inputText id="overlayBgColor" value="#{config.overlayBgColor}"/> | ||
110 | + <h:message for="overlayBgColor"/> | ||
111 | + | ||
112 | + <h:outputLabel value="overlayOpacity" for="overlayOpacity"/> | ||
113 | + <h:inputText id="overlayOpacity" value="#{config.overlayOpacity}"/> | ||
114 | + <h:message for="overlayOpacity"/> | ||
115 | + | ||
116 | + <h:outputLabel value="txtImage" for="txtImage"/> | ||
117 | + <h:inputText id="txtImage" value="#{config.txtImage}"/> | ||
118 | + <h:message for="txtImage"/> | ||
119 | + | ||
120 | + <h:outputLabel value="txtOf" for="txtOf"/> | ||
121 | + <h:inputText id="txtOf" value="#{config.txtOf}"/> | ||
122 | + <h:message for="txtOf"/> | ||
123 | + | ||
124 | + <h:outputLabel value="imageBlank" for="imageBlank"/> | ||
125 | + <h:inputText id="imageBlank" value="#{config.imageBlank}"/> | ||
126 | + <h:message for="imageBlank"/> | ||
127 | + | ||
128 | + <h:outputLabel value="imageBtnClose" for="imageBtnClose"/> | ||
129 | + <h:inputText id="imageBtnClose" value="#{config.imageBtnClose}"/> | ||
130 | + <h:message for="imageBtnClose"/> | ||
131 | + | ||
132 | + <h:outputLabel value="imageBtnNext" for="imageBtnNext"/> | ||
133 | + <h:inputText id="imageBtnNext" value="#{config.imageBtnNext}"/> | ||
134 | + <h:message for="imageBtnNext"/> | ||
135 | + | ||
136 | + <h:outputLabel value="imageBtnPrev" for="imageBtnPrev"/> | ||
137 | + <h:inputText id="imageBtnPrev" value="#{config.imageBtnPrev}"/> | ||
138 | + <h:message for="imageBtnPrev"/> | ||
139 | + | ||
140 | + <h:outputLabel value="imageLoading" for="imageLoading"/> | ||
141 | + <h:inputText id="imageLoading" value="#{config.imageLoading}"/> | ||
142 | + <h:message for="imageLoading"/> | ||
143 | + | ||
144 | + <a4j:commandButton value="Submit" reRender="lightbox,lightboxForm"/> | ||
145 | + </h:panelGrid> | ||
146 | +</h:form> | ||
147 | + | ||
148 | +<rich:insert src="/sample_1.xhtml" highlight="html"/> | ||
149 | + | ||
150 | +</body> | ||
151 | +</html> |
samples/notify-sample/pom.xml
0 → 100644
1 | +<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | ||
2 | + xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> | ||
3 | + <parent> | ||
4 | + <groupId>org.richfaces.sandbox.bernard</groupId> | ||
5 | + <artifactId>parent</artifactId> | ||
6 | + <version>3.3.4-SNAPSHOT</version> | ||
7 | + <relativePath>../pom.xml</relativePath> | ||
8 | + </parent> | ||
9 | + <modelVersion>4.0.0</modelVersion> | ||
10 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
11 | + <artifactId>notify-sample</artifactId> | ||
12 | + <packaging>war</packaging> | ||
13 | + <name>notify-sample Maven Webapp</name> | ||
14 | + <build> | ||
15 | + <finalName>${project.artifactId}</finalName> | ||
16 | + </build> | ||
17 | + <dependencies> | ||
18 | + <dependency> | ||
19 | + <groupId>org.richfaces.ui</groupId> | ||
20 | + <artifactId>richfaces-ui</artifactId> | ||
21 | + </dependency> | ||
22 | + <dependency> | ||
23 | + <groupId>org.richfaces.sandbox.ui</groupId> | ||
24 | + <artifactId>notify</artifactId> | ||
25 | + <version>${project.version}</version> | ||
26 | + </dependency> | ||
27 | + <dependency> | ||
28 | + <groupId>com.uwyn</groupId> | ||
29 | + <artifactId>jhighlight</artifactId> | ||
30 | + <version>1.0</version> | ||
31 | + </dependency> | ||
32 | + </dependencies> | ||
33 | +</project> | ||
34 | + |
1 | +package org.richfaces.sandbox.notify; | ||
2 | + | ||
3 | +import javax.faces.application.FacesMessage; | ||
4 | +import javax.faces.context.FacesContext; | ||
5 | + | ||
6 | +public class Greeter { | ||
7 | + | ||
8 | + private String greeting; | ||
9 | + private boolean nonblocking; | ||
10 | + private boolean showDetail; | ||
11 | + private boolean showSummary = true; | ||
12 | + private boolean sticky; | ||
13 | + private boolean showHistory = true; | ||
14 | + private boolean showShadow = true; | ||
15 | + private boolean showCloseButton = true; | ||
16 | + private Integer stayTime = 3000; | ||
17 | + private Integer animationSpeed = 100; | ||
18 | + private Integer interval = 2000; | ||
19 | + private Integer delay = 0; | ||
20 | + private Double nonblockingOpacity = .3; | ||
21 | + private String appearAnimation = "fade"; | ||
22 | + private String hideAnimation = "show"; | ||
23 | + private String stackDir1 = "down"; | ||
24 | + private String stackDir2 = "left"; | ||
25 | + private String stackPush = "top"; | ||
26 | + private String styleClass; | ||
27 | + private String stackStyleClass; | ||
28 | + private String title = "Sample title"; | ||
29 | + private String text = "Sample text, not too short, but not too long."; | ||
30 | + | ||
31 | + public String sayHello() { | ||
32 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_INFO, "Hello", greeting)); | ||
33 | + return "hello"; | ||
34 | + } | ||
35 | + | ||
36 | + public String warnMe() { | ||
37 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_WARN, "Stop!", greeting)); | ||
38 | + return "warn"; | ||
39 | + } | ||
40 | + | ||
41 | + public String sayError() { | ||
42 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_ERROR, "This is outrage", greeting)); | ||
43 | + return "error"; | ||
44 | + } | ||
45 | + | ||
46 | + public String sayFatal() { | ||
47 | + FacesContext.getCurrentInstance().addMessage(null, new FacesMessage(FacesMessage.SEVERITY_FATAL, "Fatality", greeting)); | ||
48 | + return "fatal"; | ||
49 | + } | ||
50 | + | ||
51 | + public String getGreeting() { | ||
52 | + return greeting; | ||
53 | + } | ||
54 | + | ||
55 | + public void setGreeting(String greeting) { | ||
56 | + this.greeting = greeting; | ||
57 | + } | ||
58 | + | ||
59 | + public boolean isNonblocking() { | ||
60 | + return nonblocking; | ||
61 | + } | ||
62 | + | ||
63 | + public void setNonblocking(boolean nonblocking) { | ||
64 | + this.nonblocking = nonblocking; | ||
65 | + } | ||
66 | + | ||
67 | + public boolean isShowDetail() { | ||
68 | + return showDetail; | ||
69 | + } | ||
70 | + | ||
71 | + public void setShowDetail(boolean showDetail) { | ||
72 | + this.showDetail = showDetail; | ||
73 | + } | ||
74 | + | ||
75 | + public void setShowSummary(boolean showSummary) { | ||
76 | + this.showSummary = showSummary; | ||
77 | + } | ||
78 | + | ||
79 | + public boolean isSticky() { | ||
80 | + return sticky; | ||
81 | + } | ||
82 | + | ||
83 | + public void setSticky(boolean sticky) { | ||
84 | + this.sticky = sticky; | ||
85 | + } | ||
86 | + | ||
87 | + public Integer getStayTime() { | ||
88 | + return stayTime; | ||
89 | + } | ||
90 | + | ||
91 | + public void setStayTime(Integer stayTime) { | ||
92 | + this.stayTime = stayTime; | ||
93 | + } | ||
94 | + | ||
95 | + public boolean isShowSummary() { | ||
96 | + return showSummary; | ||
97 | + } | ||
98 | + | ||
99 | + public String getAppearAnimation() { | ||
100 | + return appearAnimation; | ||
101 | + } | ||
102 | + | ||
103 | + public void setAppearAnimation(String appearAnimation) { | ||
104 | + this.appearAnimation = appearAnimation; | ||
105 | + } | ||
106 | + | ||
107 | + public boolean isShowHistory() { | ||
108 | + return showHistory; | ||
109 | + } | ||
110 | + | ||
111 | + public void setShowHistory(boolean showHistory) { | ||
112 | + this.showHistory = showHistory; | ||
113 | + } | ||
114 | + | ||
115 | + public boolean isShowShadow() { | ||
116 | + return showShadow; | ||
117 | + } | ||
118 | + | ||
119 | + public void setShowShadow(boolean showShadow) { | ||
120 | + this.showShadow = showShadow; | ||
121 | + } | ||
122 | + | ||
123 | + public boolean isShowCloseButton() { | ||
124 | + return showCloseButton; | ||
125 | + } | ||
126 | + | ||
127 | + public void setShowCloseButton(boolean showCloseButton) { | ||
128 | + this.showCloseButton = showCloseButton; | ||
129 | + } | ||
130 | + | ||
131 | + public Double getNonblockingOpacity() { | ||
132 | + return nonblockingOpacity; | ||
133 | + } | ||
134 | + | ||
135 | + public void setNonblockingOpacity(Double nonblockingOpacity) { | ||
136 | + this.nonblockingOpacity = nonblockingOpacity; | ||
137 | + } | ||
138 | + | ||
139 | + public String getStackDir1() { | ||
140 | + return stackDir1; | ||
141 | + } | ||
142 | + | ||
143 | + public void setStackDir1(String stackDir1) { | ||
144 | + this.stackDir1 = stackDir1; | ||
145 | + } | ||
146 | + | ||
147 | + public String getStackDir2() { | ||
148 | + return stackDir2; | ||
149 | + } | ||
150 | + | ||
151 | + public void setStackDir2(String stackDir2) { | ||
152 | + this.stackDir2 = stackDir2; | ||
153 | + } | ||
154 | + | ||
155 | + public String getStackPush() { | ||
156 | + return stackPush; | ||
157 | + } | ||
158 | + | ||
159 | + public void setStackPush(String stackPush) { | ||
160 | + this.stackPush = stackPush; | ||
161 | + } | ||
162 | + | ||
163 | + public String getStyleClass() { | ||
164 | + return styleClass; | ||
165 | + } | ||
166 | + | ||
167 | + public void setStyleClass(String styleClass) { | ||
168 | + this.styleClass = styleClass; | ||
169 | + } | ||
170 | + | ||
171 | + public Integer getAnimationSpeed() { | ||
172 | + return animationSpeed; | ||
173 | + } | ||
174 | + | ||
175 | + public void setAnimationSpeed(Integer animationSpeed) { | ||
176 | + this.animationSpeed = animationSpeed; | ||
177 | + } | ||
178 | + | ||
179 | + public String getHideAnimation() { | ||
180 | + return hideAnimation; | ||
181 | + } | ||
182 | + | ||
183 | + public void setHideAnimation(String hideAnimation) { | ||
184 | + this.hideAnimation = hideAnimation; | ||
185 | + } | ||
186 | + | ||
187 | + /** | ||
188 | + * @return the title | ||
189 | + */ | ||
190 | + public String getTitle() { | ||
191 | + return title; | ||
192 | + } | ||
193 | + | ||
194 | + /** | ||
195 | + * @param title the title to set | ||
196 | + */ | ||
197 | + public void setTitle(String title) { | ||
198 | + this.title = title; | ||
199 | + } | ||
200 | + | ||
201 | + /** | ||
202 | + * @return the text | ||
203 | + */ | ||
204 | + public String getText() { | ||
205 | + return text; | ||
206 | + } | ||
207 | + | ||
208 | + /** | ||
209 | + * @param text the text to set | ||
210 | + */ | ||
211 | + public void setText(String text) { | ||
212 | + this.text = text; | ||
213 | + } | ||
214 | + | ||
215 | + public Integer getInterval() { | ||
216 | + return interval; | ||
217 | + } | ||
218 | + | ||
219 | + public void setInterval(Integer interval) { | ||
220 | + this.interval = interval; | ||
221 | + } | ||
222 | + | ||
223 | + public Integer getDelay() { | ||
224 | + return delay; | ||
225 | + } | ||
226 | + | ||
227 | + public void setDelay(Integer delay) { | ||
228 | + this.delay = delay; | ||
229 | + } | ||
230 | + | ||
231 | + public String getStackStyleClass() { | ||
232 | + return stackStyleClass; | ||
233 | + } | ||
234 | + | ||
235 | + public void setStackStyleClass(String stackStyleClass) { | ||
236 | + this.stackStyleClass = stackStyleClass; | ||
237 | + } | ||
238 | +} |
Please
register
or
login
to post a comment